Google Trends API
Google Trends API uses /api/v1/search?engine=google_trends
API endpoint to scrape real-time results
API Parameters
Search Query
-
- Name
-
q
- Required
- Required
- Description
-
Parameter defines the search query. It can be either required or optional based on the
data_type
parameter:
data_type=TIMESERIES
- search query is required only ifcat=0
(default). It is limited to5
search queries that should be seperated by,
. For instance:Java,JavaScript,Python
.data_type=GEO_MAP
- search query is required.data_type=RELATED_QUERIES
- search query is optional.data_type=RELATED_TOPICS
- search query is optional.
Data Type
-
- Name
-
data_type
- Required
- Required
- Description
-
Parameter defines the data type you wish to search for. You have several options to choose from:
TIMESERIES
also known as Interest Over Time, used to return historical, indexed data for a given input.GEO_MAP
also known as Interest Over Region, used for geographical data.RELATED_QUERIES
corresponds to searches for queries that are related to the given input.RELATED_TOPICS
represents searches related to specific topics.
Categories
-
- Name
-
cat
- Required
- Optional
- Description
-
The parameter for category selection defaults to
0
, representing All Categories. This parameter determines the category to be used for the specified search. Please refer to the complete list of supported Google Trends Categories for more details.
-
- Name
-
region
- Required
- Optional
- Description
-
The parameter specifies the geographical region for your chosen search. It is usable only with
GEO_MAP
data_type. There are few options to consider:
COUNTRY
- selects data from country searches. Usable only with Worldwide geo value.REGION
- selects data from a states or provinces.DMA
- selects data from metros.CITY
- selects data from cities.
Localization
-
- Name
-
geo
- Required
- Optional
- Description
-
The default value for the location parameter is set to
Worldwide
, which denotes a global scope for the search. This parameter specifies the geographical area for the query search. If it is not explicitly set, the search defaults to a worldwide range. Check the full list of supported Google Trendsgeo
locations.
-
- Name
-
tz
- Required
- Optional
- Description
-
Parameter defines timezone offset (the difference in hours and minutes between a particular time zone and UTC). Could be selected from
-1439
to1439
. Default -420
.
Filters
-
- Name
-
gprop
- Required
- Optional
- Description
-
This parameter can be customized according to different search types, with each corresponding to a distinct functionality. The options include:
""
represents Web Search (Default value).images
represents Image Search.news
represents News Search.froogle
represents Google Shopping.youtube
represents YouTube Search.
-
- Name
-
time
- Required
- Optional
- Description
-
The parameter determines the time range for the data retrieval. There are a few options:
now 1-H
- data from the past hour.now 4-H
- data from the past 4 hours.now 1-d
- data from the past day.now 7-d
- data from the past 7 days.today 1-m
- data from the past 30 days.today 3-m
- data from the past 90 days.today 12-m
- data from the past 12 months.today 5-y
- data from the past 5 years.all
- All available data since 2004.
yyyy-mm-dd
. For example,2019-01-01 2019-12-31
will retrieve data for the entire year of 2019. If you want to select a specific hourly range within the past week, use the formatyyyy-mm-ddThh
. For instance,2024-07-23T21 2024-07-24T04
will retrieve data from 9PM on 2024-07-23, until 4AM on 2024-07-24. Note:tz
parameter significantly influences the results, and hourly range selections are limited to data from the previous week.
Engine
-
- Name
-
engine
- Required
- Required
- Description
-
Parameter defines an engine that will be used to retrieve real-time data. It must be set to
google_trends
.
API key
-
- Name
-
api_key
- Required
- Required
- Description
-
The
api_key
authenticates your requests. Use it as a query parameter (https://www.searchapi.io/api/v1/search?api_key=YOUR_API_KEY
) or in the Authorization header (Bearer YOUR_API_KEY
).
API Examples
Interest Over Time
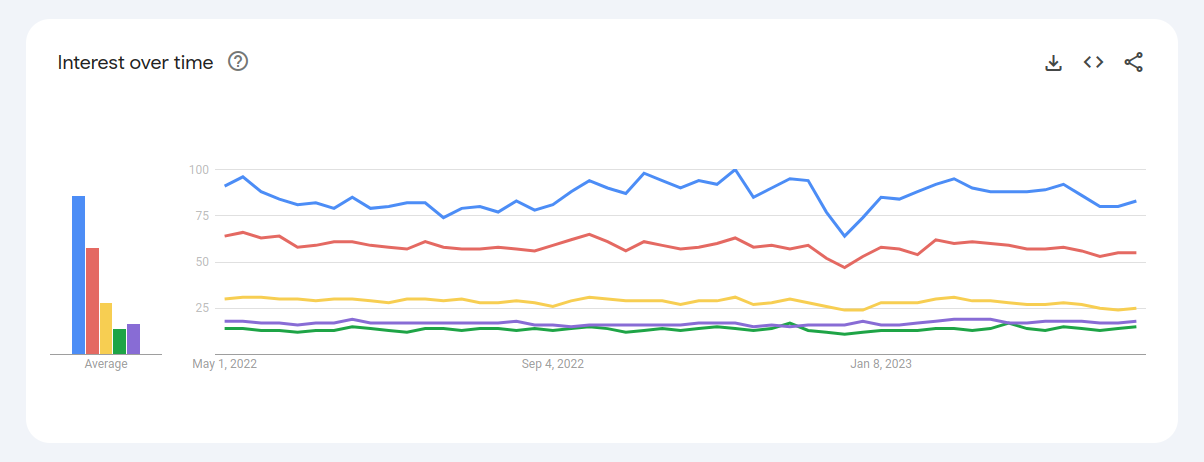
https://www.searchapi.io/api/v1/search?data_type=TIMESERIES&engine=google_trends&q=Java%2CPython%2CRuby%2CAssembly%2CJavaScript
- Python
- Node
- Ruby
- Java
- Go
- PHP
- Bash
- R
- Kotlin
- Swift
- C#
- C
- C++
- requests
import requests
url = "https://www.searchapi.io/api/v1/search"
params = {
"engine": "google_trends",
"q": "Java,Python,Ruby,Assembly,JavaScript",
"data_type": "TIMESERIES"
}
response = requests.get(url, params = params)
print(response.text)
{
"interest_over_time": {
"averages": [
{
"query": "Java",
"value": 58
},
{
"query": "Python",
"value": 85
},
{
"query": "Ruby",
"value": 17
},
{
"query": "Assembly",
"value": 13
},
{
"query": "JavaScript",
"value": 28
}
],
"timeline_data": [
{
"date": "May 1 – 7, 2022",
"timestamp": "1651363200",
"values": [
{
"query": "Java",
"value": "59",
"extracted_value": 59
},
{
"query": "Python",
"value": "87",
"extracted_value": 87
},
{
"query": "Ruby",
"value": "17",
"extracted_value": 17
},
{
"query": "Assembly",
"value": "14",
"extracted_value": 14
},
{
"query": "JavaScript",
"value": "29",
"extracted_value": 29
}
]
},
...
]
}
}
Interest by Region
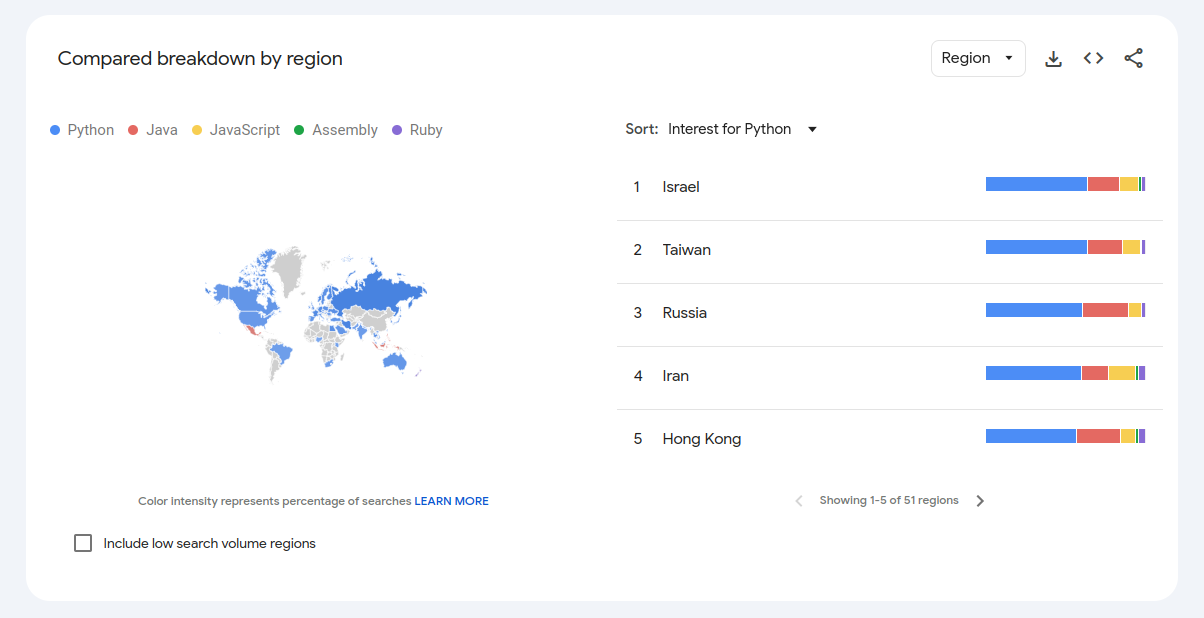
https://www.searchapi.io/api/v1/search?data_type=GEO_MAP&engine=google_trends&q=Java%2CPython%2CRuby%2CAssembly%2CJavaScript®ion=COUNTRY
- Python
- Node
- Ruby
- Java
- Go
- PHP
- Bash
- R
- Kotlin
- Swift
- C#
- C
- C++
- requests
import requests
url = "https://www.searchapi.io/api/v1/search"
params = {
"engine": "google_trends",
"q": "Java,Python,Ruby,Assembly,JavaScript",
"data_type": "GEO_MAP",
"region": "COUNTRY"
}
response = requests.get(url, params = params)
print(response.text)
{
"interest_by_region": [
{
"geo": "US",
"name": "United States",
"values": [
{
"query": "Java",
"value": "20%",
"extracted_value": 20
},
{
"query": "Python",
"value": "40%",
"extracted_value": 40
},
{
"query": "Ruby",
"value": "18%",
"extracted_value": 18
},
{
"query": "Assembly",
"value": "14%",
"extracted_value": 14
},
{
"query": "JavaScript",
"value": "8%",
"extracted_value": 8
}
]
},
...
]
}
Related Queries
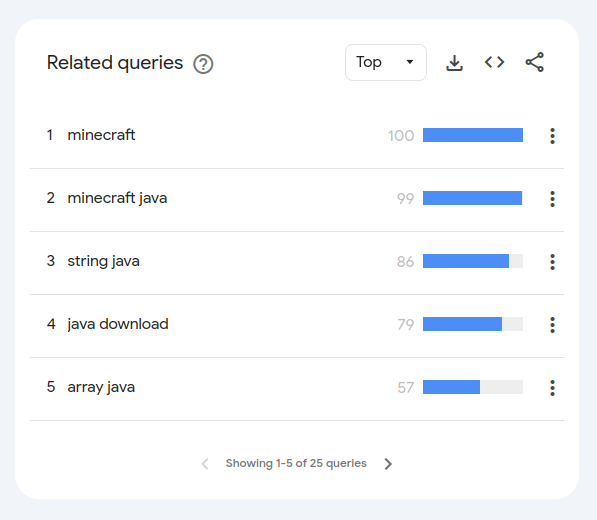
https://www.searchapi.io/api/v1/search?data_type=RELATED_QUERIES&engine=google_trends&q=Java
- Python
- Node
- Ruby
- Java
- Go
- PHP
- Bash
- R
- Kotlin
- Swift
- C#
- C
- C++
- requests
import requests
url = "https://www.searchapi.io/api/v1/search"
params = {
"engine": "google_trends",
"q": "Java",
"data_type": "RELATED_QUERIES"
}
response = requests.get(url, params = params)
print(response.text)
{
"related_queries": {
"top": [
{
"position": 1,
"query": "minecraft",
"values": "100",
"extracted_value": 100,
"link": "https://trends.google.com/trends/explore?q=minecraft&date=today+12-m"
},
{
"position": 2,
"query": "minecraft java",
"values": "99",
"extracted_value": 99,
"link": "https://trends.google.com/trends/explore?q=minecraft+java&date=today+12-m"
},
{
"position": 3,
"query": "string java",
"values": "86",
"extracted_value": 86,
"link": "https://trends.google.com/trends/explore?q=string+java&date=today+12-m"
},
...
]
}
}
Related Topics
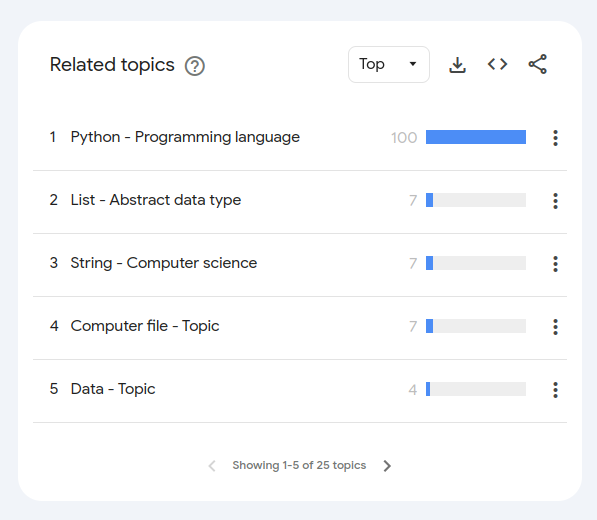
https://www.searchapi.io/api/v1/search?data_type=RELATED_TOPICS&engine=google_trends&q=Python
- Python
- Node
- Ruby
- Java
- Go
- PHP
- Bash
- R
- Kotlin
- Swift
- C#
- C
- C++
- requests
import requests
url = "https://www.searchapi.io/api/v1/search"
params = {
"engine": "google_trends",
"q": "Python",
"data_type": "RELATED_TOPICS"
}
response = requests.get(url, params = params)
print(response.text)
{
"related_topics": {
"top": [
{
"position": 1,
"id": "/m/05z1_",
"title": "Python",
"type": "Programming language",
"value": "100",
"extracted_value": 100,
"link": "https://trends.google.com/trends/explore?q=/m/05z1_&date=today+12-m"
},
{
"position": 2,
"id": "/m/01dlmc",
"title": "List",
"type": "Abstract data type",
"value": "7",
"extracted_value": 7,
"link": "https://trends.google.com/trends/explore?q=/m/01dlmc&date=today+12-m"
},
{
"position": 3,
"id": "/m/06x16",
"title": "String",
"type": "Computer science",
"value": "7",
"extracted_value": 7,
"link": "https://trends.google.com/trends/explore?q=/m/06x16&date=today+12-m"
},
...
]
}
}
Interest Over Time - Overall Category Trend

Note: The data_type=TIMESERIES
parameter cannot be used with an empty q
parameter when cat=0
.
For a list of all available categories, visit the Google Trends Categories page.
https://www.searchapi.io/api/v1/search?cat=12&data_type=TIMESERIES&engine=google_trends&geo=US
- Python
- Node
- Ruby
- Java
- Go
- PHP
- Bash
- R
- Kotlin
- Swift
- C#
- C
- C++
- requests
import requests
url = "https://www.searchapi.io/api/v1/search"
params = {
"engine": "google_trends",
"cat": "12",
"geo": "US",
"data_type": "TIMESERIES"
}
response = requests.get(url, params = params)
print(response.text)
{
"interest_over_time": {
"timeline_data": [
{
"date": "Jun 4 – 10, 2023",
"timestamp": "1685836800",
"values": [
{
"value": "83",
"extracted_value": 83
}
]
},
{
"date": "Jun 11 – 17, 2023",
"timestamp": "1686441600",
"values": [
{
"value": "92",
"extracted_value": 92
}
]
},
...
]
}
}
Related Queries - Top Search Queries Overall
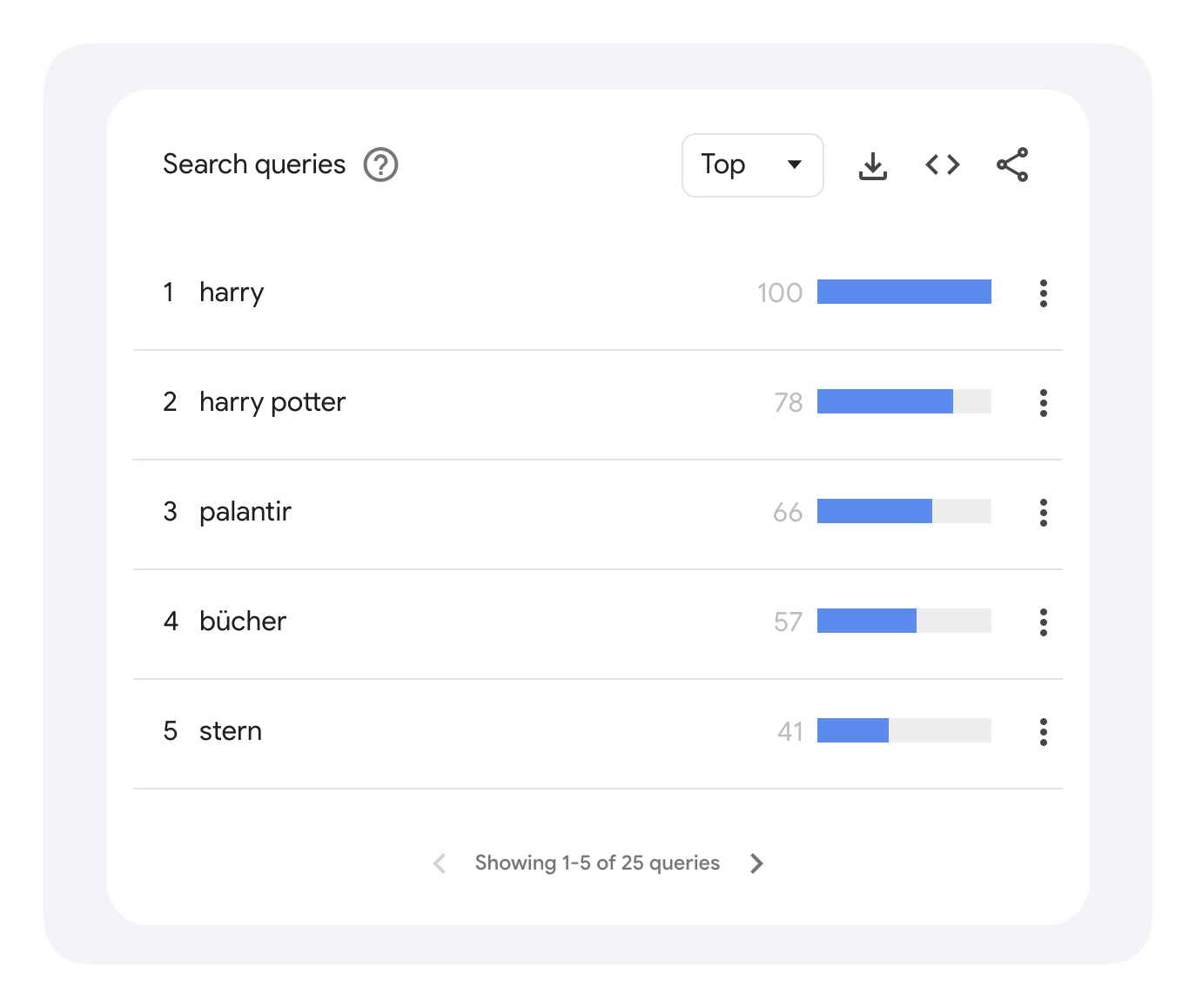
https://www.searchapi.io/api/v1/search?cat=22&data_type=RELATED_QUERIES&engine=google_trends&geo=DE&gprop=news
- Python
- Node
- Ruby
- Java
- Go
- PHP
- Bash
- R
- Kotlin
- Swift
- C#
- C
- C++
- requests
import requests
url = "https://www.searchapi.io/api/v1/search"
params = {
"engine": "google_trends",
"geo": "DE",
"cat": "22",
"gprop": "news",
"data_type": "RELATED_QUERIES"
}
response = requests.get(url, params = params)
print(response.text)
{
"related_queries": {
"top": [
{
"position": 1,
"query": "harry",
"values": "100",
"extracted_value": 100,
"link": "https://trends.google.com/trends/explore?q=harry&date=today+12-m&geo=DE"
},
{
"position": 2,
"query": "harry potter",
"values": "78",
"extracted_value": 78,
"link": "https://trends.google.com/trends/explore?q=harry+potter&date=today+12-m&geo=DE"
},
{
"position": 3,
"query": "palantir",
"values": "66",
"extracted_value": 66,
"link": "https://trends.google.com/trends/explore?q=palantir&date=today+12-m&geo=DE"
},
...
],
"rising": [
{
"position": 1,
"query": "elisa maria",
"values": "Breakout",
"extracted_value": 45350,
"link": "https://trends.google.com/trends/explore?q=elisa+maria&date=today+12-m&geo=DE"
},
{
"position": 2,
"query": "christiane f",
"values": "Breakout",
"extracted_value": 23050,
"link": "https://trends.google.com/trends/explore?q=christiane+f&date=today+12-m&geo=DE"
},
...
]
}
}
Related Topics - Top Search Topics Overall
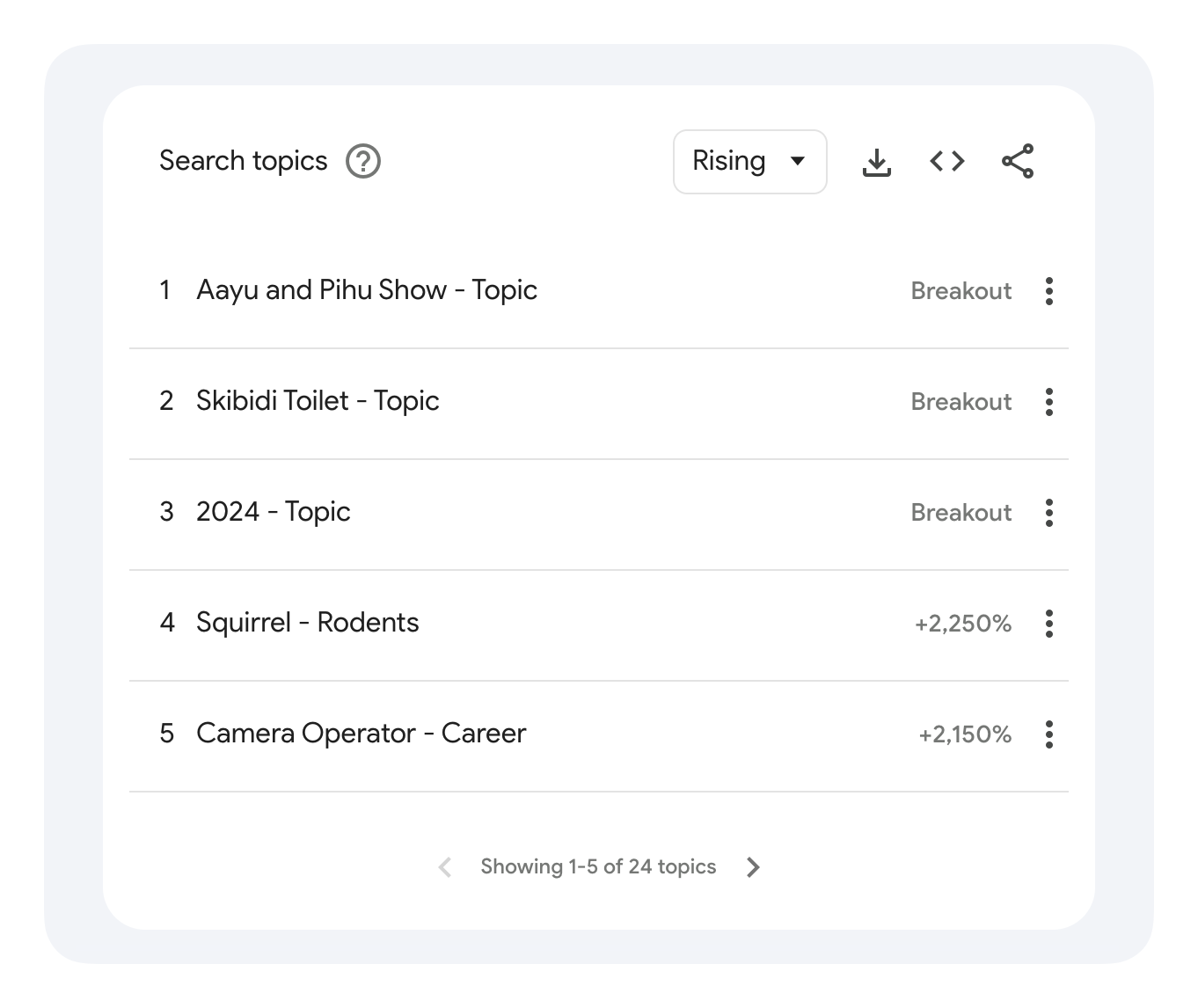
https://www.searchapi.io/api/v1/search?cat=12&data_type=RELATED_TOPICS&engine=google_trends&gprop=youtube
- Python
- Node
- Ruby
- Java
- Go
- PHP
- Bash
- R
- Kotlin
- Swift
- C#
- C
- C++
- requests
import requests
url = "https://www.searchapi.io/api/v1/search"
params = {
"engine": "google_trends",
"cat": "12",
"gprop": "youtube",
"data_type": "RELATED_TOPICS"
}
response = requests.get(url, params = params)
print(response.text)
{
"related_topics": {
"top": [
{
"position": 1,
"id": "/g/11f555cn8l",
"title": "TikTok",
"type": "Website",
"value": "100",
"extracted_value": 100,
"link": "https://trends.google.com/trends/explore?q=/g/11f555cn8l&date=today+12-m"
},
{
"position": 2,
"id": "/m/074ft",
"title": "Song",
"type": "Composition type",
"value": "34",
"extracted_value": 34,
"link": "https://trends.google.com/trends/explore?q=/m/074ft&date=today+12-m"
},
{
"position": 3,
"id": "/g/11b77b4nfc",
"title": "2023",
"type": "Topic",
"value": "33",
"extracted_value": 33,
"link": "https://trends.google.com/trends/explore?q=/g/11b77b4nfc&date=today+12-m"
},
...
],
"rising": [
{
"position": 1,
"id": "/g/11q7dqb2gg",
"title": "Aayu and Pihu Show",
"type": "Topic",
"value": "Breakout",
"extracted_value": 25150,
"link": "https://trends.google.com/trends/explore?q=/g/11q7dqb2gg&date=today+12-m"
},
{
"position": 2,
"id": "/g/11y1cqrjhh",
"title": "Skibidi Toilet",
"type": "Topic",
"value": "Breakout",
"extracted_value": 15650,
"link": "https://trends.google.com/trends/explore?q=/g/11y1cqrjhh&date=today+12-m"
},
{
"position": 3,
"id": "/g/11b77dhw02",
"title": "2024",
"type": "Topic",
"value": "Breakout",
"extracted_value": 14800,
"link": "https://trends.google.com/trends/explore?q=/g/11b77dhw02&date=today+12-m"
},
...
]
}
}