Google Maps API Documentation
The Google Maps API allows access to a wealth of map-related data. It provides details such as local business information, ratings, reviews, GPS coordinates, and more. The API can be used to search for local businesses, tourist attractions, and other points of interest based on various criteria, including keywords, types, and geographic location.
API Parameters
Search Query
-
- Name
-
q
- Required
- Required
- Description
-
Parameter defines the query you want to search. You can use anything that you would use in a regular Google Maps search.
Geographic Location
-
- Name
-
ll
- Required
- Optional
- Description
-
The parameter specifies the GPS coordinates for the location where the query (q) should be applied. It should be formatted as follows:
@latitude,longitude,zoom
For example, a properly formatted string might look like@40.7009973,-73.994778,12z
. While the zoom parameter is optional, it is advisable to use it for greater accuracy. The zoom level can range from 3z (fully zoomed out) to 21z (fully zoomed in).
Localization
-
- Name
-
google_domain
- Required
- Optional
- Description
-
The default parameter
google.com
defines the Google domain of the search. Check the full list of supported Googlegoogle_domain
domains.
-
- Name
-
hl
- Required
- Optional
- Description
-
The default parameter
en
defines the interface language of the search. Check the full list of supported Googlehl
languages.
Pagination
-
- Name
-
page
- Required
- Optional
- Description
-
This parameter indicates which page of results to return. By default, it is set to
1
.
Engine
-
- Name
-
engine
- Required
- Required
- Description
-
Parameter defines an engine that will be used to retrieve real-time data. It must be set to
google_maps
.
API key
-
- Name
-
api_key
- Required
- Required
- Description
-
The
api_key
authenticates your requests. Use it as a query parameter (https://www.searchapi.io/api/v1/search?api_key=YOUR_API_KEY
) or in the Authorization header (Bearer YOUR_API_KEY
).
API Examples
Local Results - Restaurants

https://www.searchapi.io/api/v1/search?engine=google_maps&ll=%4040.7009973%2C-73.994778%2C12z&q=Restaurants
- Python
- Node
- Ruby
- Java
- Go
- PHP
- Bash
- R
- Kotlin
- Swift
- C#
- C
- C++
- requests
import requests
url = "https://www.searchapi.io/api/v1/search"
params = {
"engine": "google_maps",
"q": "Restaurants",
"ll": "@40.7009973,-73.994778,12z"
}
response = requests.get(url, params = params)
print(response.text)
{
"search_metadata": {
"id": "search_BxR6dazVoAVkFvr3Ewvbj9GY",
"status": "Success",
"created_at": "2024-03-05T21:33:43Z",
"request_time_taken": 1.75,
"parsing_time_taken": 0.03,
"total_time_taken": 1.78,
"request_url": "https://www.google.com/maps/search/Restaurants/@40.7009973,-73.994778,12z?hl=en",
"html_url": "https://www.searchapi.io/api/v1/searches/search_BxR6dazVoAVkFvr3Ewvbj9GY.html",
"json_url": "https://www.searchapi.io/api/v1/searches/search_BxR6dazVoAVkFvr3Ewvbj9GY"
},
"search_parameters": {
"engine": "google_maps",
"q": "Restaurants",
"ll": "@40.7009973,-73.994778,12z",
"google_domain": "google.com",
"hl": "en"
},
"search_information": {
"query_displayed": "Restaurants",
"state": "Showing results for exact spelling."
},
"local_results": [
{
"position": 1,
"ludocid": "106282233335265337197",
"place_id": "ChIJueUCrBBawokRiw9LMJFm0_o",
"kgmid": "/g/1tgyxmql",
"data_id": "0x89c25a10ac02e5b9:0xfad36691304b0f8b",
"title": "O'Hara's Restaurant and Pub",
"description": "Classic Irish pub near 9-11 Memorial. Both locals & visitors come for cold pints plus burgers, wings & bar food in a neighborhood spot.",
"address": "120 Cedar St, New York, NY 10006",
"phone": "(212) 267-3032",
"price": "$$",
"price_description": "Moderately expensive",
"rating": 4.6,
"reviews": 4678,
"reviews_link": "https://search.google.com/local/reviews?placeid=ChIJueUCrBBawokRiw9LMJFm0_o&q=Restaurants&authuser=0&hl=en&gl=US",
"website": "https://www.facebook.com/OharasPubNYC/",
"domain": "facebook.com",
"gps_coordinates": {
"latitude": 40.7095008,
"longitude": -74.0126642
},
"type": "American restaurant",
"types": ["American restaurant", "Bar"],
"open_state": "Open",
"hours": "Open ⋅ Closes 1 AM",
"open_hours": {
"tuesday": "11 AM–1 AM",
"wednesday": "11 AM–1 AM",
"thursday": "11 AM–1 AM",
"friday": "11 AM–1 AM",
"saturday": "11 AM–1 AM",
"sunday": "12 PM–12 AM",
"monday": "11 AM–1 AM"
},
"extensions": [
{
"title": "Service options",
"items": [
{
"title": "Takeout",
"value": "Offers takeout"
},
...
]
},
{
"title": "Highlights",
"items": [
{
"title": "Fast service",
"value": "Has fast service"
},
...
]
},
...
],
"images": [
"https://lh5.googleusercontent.com/p/AF1QipMdOwNgxdM8jWbEy1N2UqZSQ5KTloYbOAaIPM1Q=w203-h152-k-no",
...
],
"thumbnail": "https://lh5.googleusercontent.com/p/AF1QipMdOwNgxdM8jWbEy1N2UqZSQ5KTloYbOAaIPM1Q=w122-h92-k-no"
},
...
]
}
Local Results - Hotels
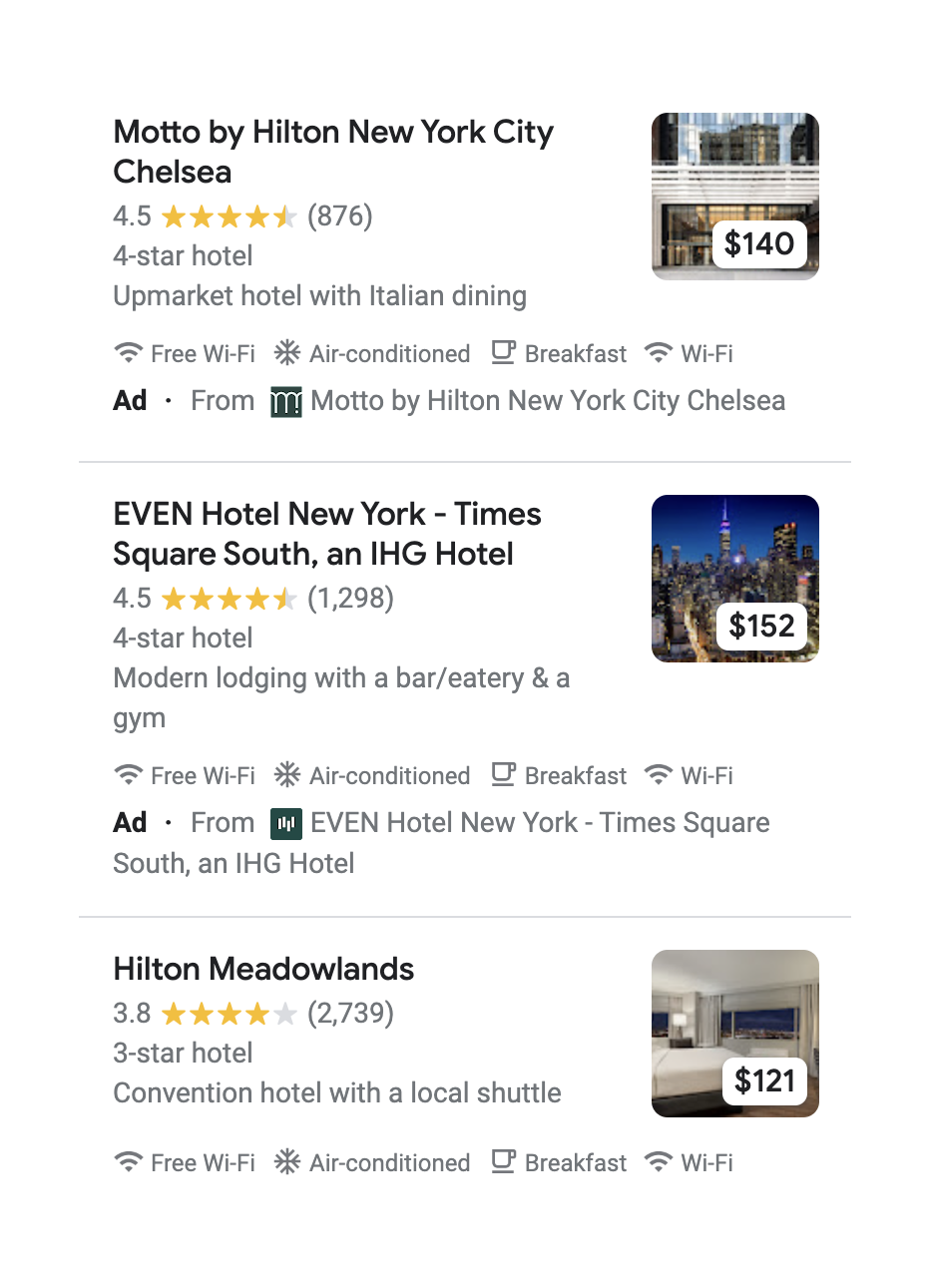
https://www.searchapi.io/api/v1/search?engine=google_maps&ll=%4040.7009973%2C-73.994778%2C12z&q=Hotels
- Python
- Node
- Ruby
- Java
- Go
- PHP
- Bash
- R
- Kotlin
- Swift
- C#
- C
- C++
- requests
import requests
url = "https://www.searchapi.io/api/v1/search"
params = {
"engine": "google_maps",
"q": "Hotels",
"ll": "@40.7009973,-73.994778,12z"
}
response = requests.get(url, params = params)
print(response.text)
{
"search_metadata": {
"id": "search_6QNVPZBKo24jcB2p8y471M9O",
"status": "Success",
"created_at": "2024-03-05T21:27:31Z",
"request_time_taken": 1.9,
"parsing_time_taken": 0.06,
"total_time_taken": 1.96,
"request_url": "https://www.google.com/maps/search/hotels/@40.7009973,-73.994778,12z?hl=en",
"html_url": "https://www.searchapi.io/api/v1/searches/search_6QNVPZBKo24jcB2p8y471M9O.html",
"json_url": "https://www.searchapi.io/api/v1/searches/search_6QNVPZBKo24jcB2p8y471M9O"
},
"search_parameters": {
"engine": "google_maps",
"q": "hotels",
"ll": "@40.7009973,-73.994778,12z",
"google_domain": "google.com",
"hl": "en"
},
"search_information": {
"query_displayed": "hotels",
"state": "Showing results for exact spelling."
},
"ads": [
{
"position": 1,
"place_id": "ChIJByUH56RZwokR0kfko980E8U",
"data_id": "0x89c259a4e7072507:0xc51334dfa3e447d2",
"title": "Motto by Hilton New York City Chelsea",
"description": "Upmarket hotel with Italian dining. Modern rooms in a high-end hotel featuring a gym, a coffee bar & an elegant Italian bar/restaurant.",
"address": "113 W 24th St, New York, NY 10001",
"price": "$140",
"hotel_stars": "4 stars",
"rating": 4.5,
"reviews": 876,
"reviews_link": "https://search.google.com/local/reviews?placeid=ChIJByUH56RZwokR0kfko980E8U&q=hotels&authuser=0&hl=en&gl=US",
"gps_coordinates": {
"latitude": 40.743728,
"longitude": -73.99313
},
"amenities": ["Free Wi-Fi", "Air-conditioned", "Breakfast", "Wi-Fi"],
"images": [
"https://lh5.googleusercontent.com/p/AF1QipOQ5vTmIjKZCcmezWz6OQfncg8zbzDww2JqR50M=w203-h135-k-no",
"https://lh5.googleusercontent.com/p/AF1QipN28SPOM-4O_YiYTiI9sBjhZUhfjfUfgJDRmm56=w203-h135-k-no",
"https://lh5.googleusercontent.com/p/AF1QipPTO_HqeryTABSqNsXlYZeDHbojnCWwKGJ2UbBu=w222-h100-k-no",
"https://lh5.googleusercontent.com/p/AF1QipPaRHtLYU6L49WPdsqQMOYwKtQkN5VRDv3l3Jgf=w203-h304-k-no"
],
"thumbnail": "https://lh5.googleusercontent.com/p/AF1QipOQ5vTmIjKZCcmezWz6OQfncg8zbzDww2JqR50M=w137-h92-k-no"
},
...
],
"local_results": [
{
"position": 1,
"ludocid": "109929969549451349998",
"place_id": "ChIJqYf1NNVXwokR-YQOOco7kcE",
"kgmid": "/m/0wfk0tt",
"data_id": "0x89c257d534f587a9:0xc1913bca390e84f9",
"title": "Hilton Meadowlands",
"description": "Convention hotel with a local shuttle. Modern high-rise conference hotel with an American restaurant & free local shuttle.",
"features": [
"This high-rise conference hotel is a 6-minute drive from Meadowlands Sports Complex and 10 miles to New York City.",
"Rooms offer classic decor and custom-designed beds, flat-screen TVs and Wi-Fi. Executive rooms provide access to the Executive Lounge's free breakfast and evening appetizers. Suites add pull-out sofas.",
"Dining options include a relaxed restaurant serving American cuisine, a lounge and a coffee shop. Other amenities include a 24/7 fitness center, a business center and over 30,000 sq ft of meeting space (including 2 ballrooms). There's also a free shuttle around the area and to Teterboro Airport."
],
"address": "Two Meadowlands Plaza, East Rutherford, NJ 07073",
"phone": "(201) 896-0500",
"price": "$121",
"hotel_stars": "3 stars",
"rating": 3.8,
"reviews": 2739,
"reviews_link": "https://search.google.com/local/reviews?placeid=ChIJqYf1NNVXwokR-YQOOco7kcE&q=hotels&authuser=0&hl=en&gl=US",
"website": "https://www.hilton.com/en/hotels/ewrsmhf-hilton-meadowlands/?SEO_id=GMB-AMER-HH-EWRSMHF&y_source=1_MTIzNjgwMy03MTUtbG9jYXRpb24ud2Vic2l0ZQ%3D%3D",
"domain": "hilton.com",
"gps_coordinates": {
"latitude": 40.8052015,
"longitude": -74.0781257
},
"type": "Hotel",
"types": ["Hotel"],
"amenities": ["Free Wi-Fi", "Air-conditioned", "Breakfast", "Wi-Fi"],
"images": [
"https://lh5.googleusercontent.com/p/AF1QipPUgX46Q1zpC-WKw-6oB9TVsbPiYkQi7O4biRPy=w203-h135-k-no",
"https://lh3.googleusercontent.com/gps-proxy/ALd4DhGwCVOo7bF8cCzuWZRTHqiWjunxJ2lcTOws3hBK9_LrJMolbpl9IJuJyqBuoFtD4m9xkIALWCzLvNHVjFHFEB37ie__py_3q1p_Z-qUbL8s3iKWV3GFny-8Knd8y_-8heQf_RLP5vUaGn6rJr3b_joXvhPkWYcsnGrNnrFXNYi-lkbfLScEp7ij=w203-h135-k-no",
"https://lh5.googleusercontent.com/p/AF1QipMUG3eTmLNiEQ4uBH82WjSEcxnI0kc8ctXuvzrp=w203-h203-k-no",
"https://lh3.googleusercontent.com/gps-proxy/ALd4DhFdh5jq6jTkXOIY5J3fzgcmIFQSZk5FOQssuFBdCg6ehiRMb98isIPJ5QUfc4ENCEzVg4kf2GHyrHfiHabzlc8qs-HVT_pZEE6WNdmK3mhf69ug3OrC24NhUSEPiGfFLn3QK1pOsdRnCEczOdluPr4_WQkxOjNeWhcW0YTL7cCQWHtIxd-8eluE=w203-h135-k-no"
],
"thumbnail": "https://lh5.googleusercontent.com/p/AF1QipPUgX46Q1zpC-WKw-6oB9TVsbPiYkQi7O4biRPy=w138-h92-k-no"
},
...
]
}
Local Results - Exact Place Details
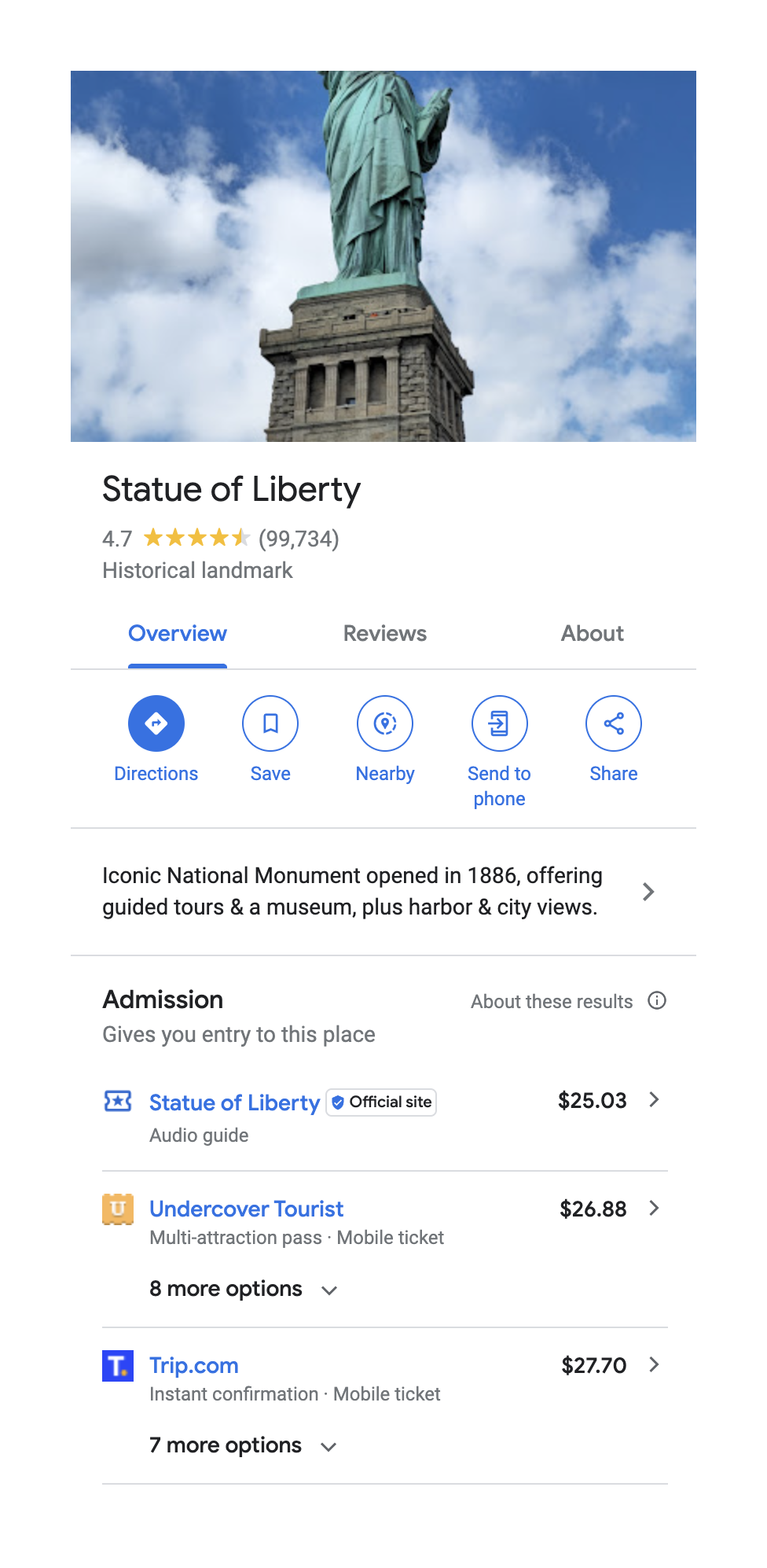
In certain cases, such as highly specific queries for well-known places, Google Maps may return a single place result.
To keep our API responses consistent, this result uses the same JSON structure as the Google Maps Place API but is included within the local_results
array as a single element.
Note that the Google Maps Place Details API provides more extensive data.
https://www.searchapi.io/api/v1/search?engine=google_maps&q=Statue+of+Liberty
- Python
- Node
- Ruby
- Java
- Go
- PHP
- Bash
- R
- Kotlin
- Swift
- C#
- C
- C++
- requests
import requests
url = "https://www.searchapi.io/api/v1/search"
params = {
"engine": "google_maps",
"q": "Statue of Liberty"
}
response = requests.get(url, params = params)
print(response.text)
{
"search_metadata": {
"id": "search_ZD40AeqmorqVsDnegkyw25lR",
"status": "Success",
"created_at": "2024-04-10T16:39:34Z",
"request_time_taken": 1.49,
"parsing_time_taken": 0.04,
"total_time_taken": 1.53,
"request_url": "https://www.google.com/maps/search/Statue+of+Liberty?hl=en",
"html_url": "https://www.searchapi.io/api/v1/searches/search_ZD40AeqmorqVsDnegkyw25lR.html",
"json_url": "https://www.searchapi.io/api/v1/searches/search_ZD40AeqmorqVsDnegkyw25lR"
},
"search_parameters": {
"engine": "google_maps",
"q": "Statue of Liberty",
"google_domain": "google.com",
"hl": "en"
},
"search_information": {
"query_displayed": "Statue of Liberty",
"state": "Showing results for original spelling."
},
"local_results": [
{
"position": 1,
"ludocid": "4667599994556318251",
"place_id": "ChIJPTacEpBQwokRKwIlDXelxkA",
"kgmid": "/m/072p8",
"data_id": "0x89c25090129c363d:0x40c6a5770d25022b",
"title": "Statue of Liberty",
"description": "American icon in New York Harbor. Iconic National Monument opened in 1886, offering guided tours & a museum, plus harbor & city views.",
"address": "New York, NY 10004",
"phone": "+1 212-363-3200",
"plus_code": "MXQ4+M5 New York",
"rating": 4.7,
"reviews": 99734,
"reviews_histogram": {
"1": 1591,
"2": 811,
"3": 3501,
"4": 14335,
"5": 79496
},
"reviews_link": "https://search.google.com/local/reviews?placeid=ChIJPTacEpBQwokRKwIlDXelxkA&q=Statue+of+Liberty&authuser=0&hl=en&gl=US",
"website": "https://www.nps.gov/stli/index.htm",
"domain": "nps.gov",
"gps_coordinates": {
"latitude": 40.6892494,
"longitude": -74.04450039999999
},
"type": "Historical landmark",
"types": ["Historical landmark", "Monument", "Tourist attraction"],
"open_state": "Open",
"hours": "Open ⋅ Closes 5 PM",
"open_hours": {
"wednesday": "9 AM–5 PM",
"thursday": "9 AM–5 PM",
"friday": "9 AM–5 PM",
"saturday": "9 AM–5 PM",
"sunday": "9 AM–5 PM",
"monday": "9 AM–5 PM",
"tuesday": "9 AM–5 PM"
},
"thumbnail": "https://lh5.googleusercontent.com/p/AF1QipNOkjsKcMBL_Fia95bCQvwISPZBNG_Addfw3AYm=w86-h114-k-no",
"images": [
{
"title": "All",
"thumbnail": "https://lh5.googleusercontent.com/p/AF1QipNOkjsKcMBL_Fia95bCQvwISPZBNG_Addfw3AYm=w224-h298-k-no"
},
...
],
"extensions": [
{
"title": "Accessibility",
"items": [
{
"title": "Wheelchair accessible entrance",
"value": "Has wheelchair accessible entrance"
},
{
"title": "Wheelchair accessible parking lot",
"value": "Has wheelchair accessible parking lot"
}
]
},
{
"title": "Planning",
"items": [
{
"title": "Getting tickets in advance recommended",
"value": "Getting tickets in advance recommended"
}
]
},
...
],
"admissions": [
{
"title": "Statue of Liberty",
"favicon": "https://www.gstatic.com/ads-travel/ttd/official_admission_32x32.png",
"options": [
{
"link": "https://www.cityexperiences.com/new-york/city-cruises/statue/new-york-pedestal-reserve/",
"price": "$25.03",
"extracted_price": 25.03,
"official_site": true
}
]
},
...
],
"experiences": [
{
"kgmid": "/m/072p8",
"title": "Statue of Liberty Express Tour",
"link": "https://www.exp1.com/new-york-tours/statue-of-liberty-express-tour/?utm_source=gtd&utm_medium=gtd&utm_campaign=mlw",
"rating": 5,
"reviews": 98,
"price": "$69",
"extracted_price": 69,
"source": "ExperienceFirst",
"duration": "3h",
"favicon": "https://encrypted-tbn2.gstatic.com/faviconV2?url=https://www.exp1.com/&client=DESTINATION_EXPLORE&type=FAVICON&size=32&fallback_opts=TYPE,SIZE,URL&nfrp=1",
"images": [
"https://encrypted-tbn3.gstatic.com/images?q=tbn:ANd9GcSDuKvUobu27sqdnIiW7KpBzK5HpsIpPeYD6rNPrQIozkPZsFob",
...
]
},
...
],
"questions_and_answers": {
"question": {
"user": {
"name": "People are asking"
},
"text": "Are people allowed in the Statue of Liberty's crown?",
"date": "Frequently searched on Google",
"language": "en"
},
"answer": {
"user": {
"name": "Arka Chowdhury",
"link": "https://www.google.com/maps/contrib/102224277231379136438",
"thumbnail": "https://lh3.googleusercontent.com/a-/ALV-UjU6PTxXrnaz7c6iwOENSp3vfTSoUr56VGIVXeIzPsRa-fqr1tb7=s120-c-rp-mo-ba3-br100"
},
"text": "Yes, you have to book for crown access before hand online.",
"date": "6 years ago",
"language": "en"
},
"total_answers": 221
},
"at_this_place": {
"categories": [
{
"title": "Food & Drink",
"places_count": 1
},
...
],
"local_results": [
{
"position": 1,
"ludocid": "6610159946571001492",
"kgmid": "/g/12m9h99dz",
"data_id": "0x89c2508ed4dd0f3b:0x5bbc017303e12e94",
"title": "Statue of Liberty Crown Cafe",
"gps_coordinates": {
"latitude": 40.6899925,
"longitude": -74.04629349999999
},
"rating": 4.1,
"reviews": 2016,
"reviews_histogram": {
"1": 121,
"2": 131,
"3": 268,
"4": 490,
"5": 1006
},
"price": "$$",
"address": "Liberty Island, Manhattan, NY 10004",
"open_state": "Open ⋅ Closes 5 PM",
"open_hours": {
"wednesday": "9:30 AM–5 PM",
"thursday": "9:30 AM–5 PM",
"friday": "9:30 AM–5 PM",
"saturday": "9:30 AM–5 PM",
"sunday": "9:30 AM–5 PM",
"monday": "9:30 AM–5 PM",
"tuesday": "9:30 AM–5 PM"
},
"type": "Restaurant",
"thumbnail": "https://lh5.googleusercontent.com/p/AF1QipOOznNuT0LH11169AidPcYJ77kVYGxTRNto3gy4=w86-h86-n-k-no"
},
...
]
},
"review_results": {
"summaries": [
"\"Soda, water, juices, beers and wines help rinse the good food down.\"",
"\"An awesome place to eat big hot dogs,, big pizzas, and big fries.\"",
"\"Rude people on security line no trash cans inside waste of money and energy\""
],
"reviews": [
{
"username": "Alexandre Santos e Alves",
"rating": 5,
"contributor_id": "108447521185407051177",
"description": "The Statue of Liberty is a historical icon, so it is a must-see for anyone going to New York. A symbol of freedom that captivates visitors the moment they see it.\n\nThe experience of arriving at the island by boat, with stunning views of New York City and the statue itself, is exhilarating. Upon arriving on the island, it is possible to appreciate the grandeur and beauty of this historical monument up close.\n\nEverything is very organized, the team is very prepared and attentive, and the museum provides a lot of information about the construction of the statue.\n\nIn short, the Statue of Liberty is a jewel of New York City and a powerful reminder of the ideals of freedom and hope.",
"link": "https://www.google.com/maps/reviews/data=!4m8!14m7!1m6!2m5!1sChdDSUhNMG9nS0VJQ0FnSUQ5cEpfVnBBRRAB!2m1!1s0x0:0x40c6a5770d25022b!3m1!1s2@1:CIHM0ogKEICAgID9pJ_VpAE%7CCgwI6qjGrwYQgLHrwAI%7C?hl=en-US",
"date": "4 weeks ago",
"images": [
"https://lh5.googleusercontent.com/p/AF1QipOOm8-dt__LAyy29nBOKyTEsDHONQEX42mbqAu0=w150-h150-k-no-p",
...
]
},
...
]
},
"people_also_search_for": [
{
"position": 1,
"data_id": "0x0:0x35c71b135cfce478",
"ludocid": "3875095774354007160",
"title": "Balto Statue",
"gps_coordinates": {
"latitude": 40.7699742,
"longitude": -73.9710197
},
"rating": 4.7,
"reviews": 2150,
"types": [
"Tourist attraction",
"Historical landmark",
"Scenic spot",
"Sculpture"
],
"thumbnail": "https://lh5.googleusercontent.com/p/AF1QipOdJpw3dZHu4CVOaKbWViIxuNBkNYzHkezFGkLD=w156-h156-n-k-no"
},
...
],
"popular_times": {
"live": {
"info": "A little busy",
"typical_time_spent": "People typically spend up to 2 hours here"
},
"chart": {
"sunday": [
{
"time": "6 AM",
"busyness_score": 0
},
...
],
...
}
}
}
]
}