Google Shopping API
Google Shopping API uses /api/v1/search?engine=google_shopping
API endpoint to scrape real-time results.
The Google Shopping API delivers a dynamic product search and comparison service. It compiles product data from a multitude of biggest merchants across the globe, categorizes similar products, and presents them in accordance with each user's search query.
API Parameters
Search Query
-
- Name
-
q
- Required
- Required
- Description
-
Parameter defines the query you want to search. You can use anything that you would use in a regular Google search.
Geographic Location
-
- Name
-
location
- Required
- Optional
- Description
-
Parameter defines from where you want the search to originate. If several locations match the location requested, we'll pick the most popular one. Head to the Locations API if you need more precise control.
-
- Name
-
uule
- Required
- Optional
- Description
-
Parameter is the Google encoded location you want to use for the search. SearchApi automatically generated the
uule
parameter when you use thelocation
parameter but we allow you to overwrite it directly.uule
andlocation
parameters can't be used together.
Localization
-
- Name
-
google_domain
- Required
- Optional
- Description
-
The default parameter
google.com
defines the Google domain of the search. Check the full list of supported Googlegoogle_domain
domains.
-
- Name
-
gl
- Required
- Optional
- Description
-
The default parameter
us
defines the country of the search. Check the full list of supported Googlegl
countries.
-
- Name
-
hl
- Required
- Optional
- Description
-
The default parameter
en
defines the interface language of the search. Check the full list of supported Googlehl
languages.
Filters
-
- Name
-
tbs
- Required
- Optional
- Description
-
This parameter restricts results to URLS based on encoded values. For instance,
mr:1,local_avail:1,ss:55
would filter products by nearby results with 55 miles radius. When tbs parameter is used, other filters are ignored.
-
- Name
-
sort_by
- Required
- Optional
- Description
-
This parameter controls the sort order. By default it returns products sorted by relevance. There are few more options to sort products:
relevance
- Relevance (default).review_score
- Review score.price_low_to_high
- Price: low to high.price_high_to_low
- Price: high to low.
tbs
parameter.
-
- Name
-
price_min
- Required
- Optional
- Description
-
This parameter controls the minimum price of the products returned. For instance -
100
value would return products with a minimum price of 100. Note: minimum price can't be higher than maximum price and this parameter is not compatible with a customtbs
parameter.
-
- Name
-
price_max
- Required
- Optional
- Description
-
This parameter controls the maximum price of the products returned. For instance -
2.50
value would return products with a maximum price of 2.50. Note: maximum price can't be lower than minimum price and this parameter is not compatible with a customtbs
parameter.
-
- Name
-
condition
- Required
- Optional
- Description
-
This parameter filters product condition. There are two options:
new
- for New products andused
- for Used items. Note: this parameter is not compatible with a customtbs
parameter.
Pagination
-
- Name
-
num
- Required
- Optional
- Description
-
This parameter specifies the number of results to display per page. By default, it is set to
60
. Use in combination with thepage
parameter to implement pagination functionality. Max num per page is100
, min -1
Edge case: Settingnum=10
does not work as intended. Google Shopping page returns60
shopping results per page instead of10
.
-
- Name
-
page
- Required
- Optional
- Description
-
This parameter indicates which page of results to return. By default, it is set to
1
. Use in combination with the num parameter to implement pagination.
Engine
-
- Name
-
engine
- Required
- Required
- Description
-
Parameter defines an engine that will be used to retrieve real-time data. It must be set to
google_shopping
.
API key
-
- Name
-
api_key
- Required
- Required
- Description
-
The
api_key
authenticates your requests. Use it as a query parameter (https://www.searchapi.io/api/v1/search?api_key=YOUR_API_KEY
) or in the Authorization header (Bearer YOUR_API_KEY
).
API Examples
Full Response

https://www.searchapi.io/api/v1/search?engine=google_shopping&location=California%2CUnited+States&q=PS5
- Python
- Node
- Ruby
- Java
- Go
- PHP
- Bash
- R
- Kotlin
- Swift
- C#
- C
- C++
- requests
import requests
url = "https://www.searchapi.io/api/v1/search"
params = {
"engine": "google_shopping",
"q": "PS5",
"location": "California,United States"
}
response = requests.get(url, params = params)
print(response.text)
{
"search_metadata": {
"id": "search_81zxXmPv3DdnFwQA3VdOanD5",
"status": "Success",
"created_at": "2023-07-05T15:22:30Z",
"request_time_taken": 1.62,
"parsing_time_taken": 0.27,
"total_time_taken": 1.89,
"request_url": "https://www.google.com/search?q=PS5&oq=PS5&gl=us&hl=en&tbm=shop&tbs=mr:1&uule=w+CAIQICIYQ2FsaWZvcm5pYSxVbml0ZWQgU3RhdGVz&ie=UTF-8",
"html_url": "https://www.searchapi.io/api/v1/searches/search_81zxXmPv3DdnFwQA3VdOanD5.html",
"json_url": "https://www.searchapi.io/api/v1/searches/search_81zxXmPv3DdnFwQA3VdOanD5"
},
"search_parameters": {
"engine": "google_shopping",
"q": "PS5",
"location": "California,United States",
"location_used": "California,United States",
"google_domain": "google.com",
"hl": "en",
"gl": "us"
},
"search_information": {
"query_displayed": "PS5",
"page": 1,
"detected_location": "California"
},
"filters": [
{
"type": "Show only",
"options": [
{
"text": "Buy on Google",
"tbs": "mr:1,buy:g"
},
{
"text": "Available nearby",
"tbs": "mr:1,local_avail:1,ss:55"
},
{
"text": "On sale",
"tbs": "mr:1,sales:1"
}
]
},
{
"type": "Price",
"options": [
{
"text": "Up to $150",
"tbs": "mr:1,price:1,ppr_max:150"
},
{
"text": "$150 – $350",
"tbs": "mr:1,price:1,ppr_min:150,ppr_max:350"
},
{
"text": "$350 – $500",
"tbs": "mr:1,price:1,ppr_min:350,ppr_max:500"
},
{
"text": "$500 – $700",
"tbs": "mr:1,price:1,ppr_min:500,ppr_max:700"
},
{
"text": "Over $700",
"tbs": "mr:1,price:1,ppr_min:700"
}
]
},
{
"type": "Color",
"options": [
{
"text": "White",
"tbs": "mr:1,pdtr0:1717142%7C1717158"
},
{
"text": "Black",
"tbs": "mr:1,pdtr0:1717142%7C1717146"
},
{
"text": "Purple",
"tbs": "mr:1,pdtr0:1717142%7C3564777"
},
{
"text": "Blue",
"tbs": "mr:1,pdtr0:1717142%7C1717147"
}
...
]
}
...
],
"shopping_ads": [
{
"position": 1,
"block_position": "top",
"title": "Sony Video Games & Consoles | White, Ps5 With Controller | Color: White | Size: Os | Julious_Moore's Closet",
"link": "https://www.google.com/aclk?...",
"seller": "Poshmark",
"price": "$500.00",
"extracted_price": 500,
"image": "https://encrypted-tbn3.gstatic.com/shopping?q=tbn:ANd9GcQLKeZgXNpSjfP8cpDduhNyjhGt4jyHu-yCfsgSDoyOl7dQx8JTvgZwphBt8O2t24BpR0igiOUuhqag9BGafeyhsoVABfyyhM74U8LqBgA&usqp=CAE"
},
{
"position": 2,
"block_position": "top",
"title": "PlayStation 5 Digital Edition Console - Sony PS5 Console",
"link": "https://www.google.com/aclk?...",
"seller": "PlayStation",
"price": "$399.99",
"extracted_price": 399.99,
"image": "https://encrypted-tbn0.gstatic.com/shopping?q=tbn:ANd9GcR4KKGxDXG0ekvyaR2dtvuHANh3Xcw0w7Pk1DsUd9Fn6l7uBMtcUIVE-gbUM18zG8OwpF7lvhht1CTDq687ugzE_yDQO1ErTGWKE6XQwrGRkqVpAEabuHT78w&usqp=CAE"
},
{
"position": 3,
"block_position": "top",
"title": "Sony PlayStation 5 Digital Edition Console",
"link": "https://www.google.com/aclk?...",
"seller": "GameStop",
"rating": 4.5,
"reviews": 84610,
"price": "$399.99",
"extracted_price": 399.99,
"image": "https://encrypted-tbn3.gstatic.com/shopping?q=tbn:ANd9GcREtCJG5fagzfc14mBQCMXhV2JNqr-ui9Ak0YciG85OCYzHJAXrIVE-5j2lgxewMLVNwS7Rz575PDGU1TnEexd5UIvEqeoGuKqPnaiB5tFMiT3kjhFR4K1KpQ&usqp=CAE"
},
{
"position": 4,
"block_position": "top",
"title": "Playstation 5 GOW Ragnarok Bundle - new",
"link": "https://www.google.com/aclk?...",
"seller": "GameFly",
"price": "$499.99",
"extracted_price": 499.99,
"image": "https://encrypted-tbn3.gstatic.com/shopping?q=tbn:ANd9GcQJlFVUc6UTOx1PP9xgVA17JfHc5iTyxnyJRNPUxp2W6DG8EqoZYZ2WiWyOGyH70x4pt-HvQ4tyIqzhMeUfV7bQi0UQAhldaNGU_bvhiyCWyubHCF_lcU5N1Q&usqp=CAE"
},
{
"position": 5,
"block_position": "top",
"title": "Sony Playstation 5 Console - Call Of Duty Modern Warfare Ii Bundle - 1000030612",
"link": "https://www.google.com/aclk?...",
"seller": "Dell",
"rating": 4.5,
"reviews": 84610,
"price": "$539.99",
"extracted_price": 539.99,
"image": "https://encrypted-tbn3.gstatic.com/shopping?q=tbn:ANd9GcQqe63al49SCTetjusdwSpG4dAktIrX-nAa4zLXwRfn-O6NLokvqTzHfGDqs65gnfI0hcFhnSg9s9nV2vYug--0_Qe6DxdVhEJXsFGRr4LDZZadt7BAZo_o&usqp=CAE"
},
{
"position": 6,
"block_position": "top",
"title": "Sony PlayStation 5 Console",
"link": "https://www.google.com/aclk?...",
"seller": "BuyDirect & Save",
"rating": 4.5,
"reviews": 84610,
"price": "$699.00",
"extracted_price": 699,
"image": "https://encrypted-tbn1.gstatic.com/shopping?q=tbn:ANd9GcQvKHf5qp9dNTe67Jkn-7U9h8JBrc_5wl1ByiRU6KMq8josN3whZLDokxjtCTBZJi6szV7epgv1-BoXPknM0euVLioNJNV2uIeZbe6vy8phLUwUdE-xape4EQ&usqp=CAE"
}
...
],
"shopping_results": [
{
"position": 1,
"product_id": "8039622388823297908",
"title": "PlayStation 5 Digital Edition 825gb - White",
"link": "https://www.walmart.com/ip/Sony-PlayStation-5-Digital-Edition-Video-Game-Consoles/1601982539?wmlspartner=wlpa&selectedSellerId=0&rct=j&q=&esrc=s&sa=U&ved=0ahUKEwiOo_--7_f_AhWYLUQIHdFuABYQguUECKcQ&usg=AOvVaw2I0URFrfTa1fPBjTLE4C15",
"product_link": "https://www.google.com/shopping/product/8039622388823297908?q=PS5&ie=UTF-8&uule=w+CAIQICIYQ2FsaWZvcm5pYSxVbml0ZWQgU3RhdGVz&oq=PS5&gl=us&hl=en&prds=eto:11629306987267378527_0,pid:2168478264439240845,rsk:PC_9780189300843342177&sa=X&ved=0ahUKEwiOo_--7_f_AhWYLUQIHdFuABYQ8gIInhAoAA",
"source": "Walmart",
"comparisons": "Compare prices from 10+ stores",
"comparisons_link": "https://www.google.com/shopping/product/8039622388823297908/offers?q=PS5&ie=UTF-8&uule=w+CAIQICIYQ2FsaWZvcm5pYSxVbml0ZWQgU3RhdGVz&oq=PS5&gl=us&hl=en&prds=eto:11629306987267378527_0,pid:2168478264439240845,rsk:PC_9780189300843342177&sa=X&ved=0ahUKEwiOo_--7_f_AhWYLUQIHdFuABYQ3q4ECMQQ",
"price": "$399.00",
"extracted_price": 399,
"rating": 4.7,
"reviews": 84610,
"delivery": "Free delivery & Free 90-day returns",
"badge": "Top Quality Store",
"extensions": [
"4K Capable",
"White",
"Wi-Fi"
],
"durability": "Used",
"merchant": {
"rating": 4.4,
"reviews": 1000,
"link": "https://www.google.com/shopping/ratings/account/metrics?q=walmart.com&c=US&v=19&hl=en&rct=j&q=&esrc=s&sa=U&ved=0ahUKEwiOo_--7_f_AhWYLUQIHdFuABYQ9-wCCK8Q&usg=AOvVaw310eu3EHmZlzb0J9D1K053"
},
"thumbnail": "https://encrypted-tbn3.gstatic.com/shopping?q=tbn:ANd9GcRZCoChIvlmWoNq_liQ0Vb62nfQz48O1w-tqrScv1r5R5V92qkBGry2U9S-SOhdIgZwlemY139CrTgqC7OF1fY8PQO5VzeG1iMhP3i_mDQ&usqp=CAE"
},
{
"position": 2,
"product_id": "16230039729797264158",
"title": "Sony PlayStation 5 Console Disc PS5",
"link": "https://direct.playstation.com/en-us/buy-consoles/playstation5-console&rct=j&q=&esrc=s&sa=U&ved=0ahUKEwiOo_--7_f_AhWYLUQIHdFuABYQguUECNQQ&usg=AOvVaw3sgsyceCRSf2WvXPdEav3s",
"product_link": "https://www.google.com/shopping/product/16230039729797264158?q=PS5&ie=UTF-8&uule=w+CAIQICIYQ2FsaWZvcm5pYSxVbml0ZWQgU3RhdGVz&oq=PS5&gl=us&hl=en&prds=eto:9840307321043010602_0,pid:277778081304797025,rsk:PC_9780189300843342177&sa=X&ved=0ahUKEwiOo_--7_f_AhWYLUQIHdFuABYQ8gIIyxAoAA",
"source": "PlayStation",
"comparisons": "Compare prices from 10+ stores",
"comparisons_link": "https://www.google.com/shopping/product/16230039729797264158/offers?q=PS5&ie=UTF-8&uule=w+CAIQICIYQ2FsaWZvcm5pYSxVbml0ZWQgU3RhdGVz&oq=PS5&gl=us&hl=en&prds=eto:9840307321043010602_0,pid:277778081304797025,rsk:PC_9780189300843342177&sa=X&ved=0ahUKEwiOo_--7_f_AhWYLUQIHdFuABYQ3q4ECNwQ",
"price": "$499.99",
"extracted_price": 499.99,
"rating": 4.7,
"reviews": 84610,
"delivery": "Free delivery & Free 30-day returns",
"badge": "Top Quality Store",
"extensions": [
"4K Capable",
"White",
"Wi-Fi"
],
"merchant": {
"rating": 4.5,
"reviews": 3200,
"link": "https://www.google.com/shopping/ratings/account/metrics?q=playstation.com&c=US&v=19&hl=en&rct=j&q=&esrc=s&sa=U&ved=0ahUKEwiOo_--7_f_AhWYLUQIHdFuABYQ9-wCCNsQ&usg=AOvVaw3MkOz7v6vslpqEw95jZ1Dg"
},
"thumbnail": "https://encrypted-tbn3.gstatic.com/shopping?q=tbn:ANd9GcQ2u78RdDkSiXPOwKnuBIP3-kEqG39RkcZUn-g_iJI86g_MBDhIhaB9AksV0jGWkAFeNCJuUaKbabfgKm727MFGt5ubDn-0WBV8wlSsWqtS1sYAKrMKy9oRaw&usqp=CAE"
},
{
"position": 3,
"product_id": "4887235756540435899",
"title": "Sony PlayStation 5 - Digital Edition",
"link": "https://www.gamestop.com/consoles-hardware/playstation-5/consoles/products/sony-playstation-5-digital-edition-console/225171.html&rct=j&q=&esrc=s&sa=U&ved=0ahUKEwiOo_--7_f_AhWYLUQIHdFuABYQguUECO0Q&usg=AOvVaw0zTzA7cCgs_fPzbTNxV6GJ",
"product_link": "https://www.google.com/shopping/product/4887235756540435899?q=PS5&ie=UTF-8&uule=w+CAIQICIYQ2FsaWZvcm5pYSxVbml0ZWQgU3RhdGVz&oq=PS5&gl=us&hl=en&prds=eto:1950949305448862466_0,pid:9775745750560217909,rsk:PC_9780189300843342177&sa=X&ved=0ahUKEwiOo_--7_f_AhWYLUQIHdFuABYQ8gII5BAoAA",
"source": "GameStop",
"comparisons": "Compare prices from 10+ stores",
"comparisons_link": "https://www.google.com/shopping/product/4887235756540435899/offers?q=PS5&ie=UTF-8&uule=w+CAIQICIYQ2FsaWZvcm5pYSxVbml0ZWQgU3RhdGVz&oq=PS5&gl=us&hl=en&prds=eto:1950949305448862466_0,pid:9775745750560217909,rsk:PC_9780189300843342177&sa=X&ved=0ahUKEwiOo_--7_f_AhWYLUQIHdFuABYQ3q4ECPUQ",
"price": "$399.99",
"extracted_price": 399.99,
"rating": 4.7,
"reviews": 84610,
"tag": "GREAT PICKThis product and store are highly rated, and the price is competitive.",
"delivery": "Free delivery by Jul 7 & Free 30-day returns",
"badge": "Top Quality Store",
"extensions": [
"4K Capable",
"White",
"Wi-Fi"
],
"merchant": {
"rating": 4.5,
"reviews": 356,
"link": "https://www.google.com/shopping/ratings/account/metrics?q=gamestop.com&c=US&v=19&hl=en&rct=j&q=&esrc=s&sa=U&ved=0ahUKEwiOo_--7_f_AhWYLUQIHdFuABYQ9-wCCPQQ&usg=AOvVaw0nyn8xAJWRVIQSDnFOMAlT"
},
"thumbnail": "https://encrypted-tbn3.gstatic.com/shopping?q=tbn:ANd9GcTBXMwHJGw_cTIJFUl8uIw-QookG2OIZKH5i4PTB831_avE-N8ENQBWJ2oN3vZx-iAIAIXYlgLrwTxDVTkDmDrUSaThYU2WM6r2jAzh14GbIdKxKpReYhSIAA&usqp=CAE"
},
{
"position": 4,
"product_id": "9015061063376159526",
"title": "PS5 Digital Edition – God of War Ragnarök Bundle",
"link": "https://www.amazon.com/PS5-Digital-Ragnar%C3%B6k-Bundle-PlayStation-5/dp/B0BHBWSJN3?source=ps-sl-shoppingads-lpcontext&ref_=fplfs&psc=1&smid=ATVPDKIKX0DER&rct=j&q=&esrc=s&sa=U&ved=0ahUKEwiOo_--7_f_AhWYLUQIHdFuABYQguUECIER&usg=AOvVaw2SnvNrLiEA-ruUAQlxBfyK",
"product_link": "https://www.google.com/shopping/product/9015061063376159526?q=PS5&ie=UTF-8&uule=w+CAIQICIYQ2FsaWZvcm5pYSxVbml0ZWQgU3RhdGVz&oq=PS5&gl=us&hl=en&prds=eto:6029260381273122139_0,pid:18337215447371151693,rsk:PC_11266645096928914772&sa=X&ved=0ahUKEwiOo_--7_f_AhWYLUQIHdFuABYQ8gII-xAoAA",
"source": "Amazon.com",
"price": "$459.00",
"extracted_price": 459,
"rating": 4.8,
"reviews": 40104,
"delivery": "Free delivery",
"badge": "Top Quality Store",
"extensions": [
"4K Capable"
],
"merchant": {
"rating": 4.5,
"reviews": 2900,
"link": "https://www.google.com/shopping/ratings/account/metrics?q=amazon.com&c=US&v=19&hl=en&rct=j&q=&esrc=s&sa=U&ved=0ahUKEwiOo_--7_f_AhWYLUQIHdFuABYQ9-wCCIgR&usg=AOvVaw1iVHUP-WKDjSj1asAtMY4t"
},
"thumbnail": "https://encrypted-tbn3.gstatic.com/shopping?q=tbn:ANd9GcQYeRipcv2FRjYNv5eJNemTpjoE8u9RL60wABdHYtlVbfGY_PzGXrQHBwfYyBvVVN4s68S2d_El9SeGWkTBwur1hJNSvTyOpR1LlAUCDGymsve4VOsLuZ16PXg&usqp=CAE"
},
{
"position": 5,
"product_id": "15267646793941310045",
"title": "Sony PlayStation 5 Console - Disc version, White",
"link": "https://www.mercari.com/us/item/m46043208648/&rct=j&q=&esrc=s&sa=U&ved=0ahUKEwiOo_--7_f_AhWYLUQIHdFuABYQguUECJUR&usg=AOvVaw2wVi_ftRBgj1GsLY-_bscD",
"product_link": "https://www.google.com/shopping/product/15267646793941310045?q=PS5&ie=UTF-8&uule=w+CAIQICIYQ2FsaWZvcm5pYSxVbml0ZWQgU3RhdGVz&oq=PS5&gl=us&hl=en&prds=eto:17570281275177271302_0,pid:7862312007813342423,rsk:PC_9780189300843342177&sa=X&ved=0ahUKEwiOo_--7_f_AhWYLUQIHdFuABYQ8gIIjhEoAA",
"source": "Mercari",
"comparisons": "Compare prices from 5+ stores",
"comparisons_link": "https://www.google.com/shopping/product/15267646793941310045/offers?q=PS5&ie=UTF-8&uule=w+CAIQICIYQ2FsaWZvcm5pYSxVbml0ZWQgU3RhdGVz&oq=PS5&gl=us&hl=en&prds=eto:17570281275177271302_0,pid:7862312007813342423,rsk:PC_9780189300843342177&sa=X&ved=0ahUKEwiOo_--7_f_AhWYLUQIHdFuABYQ3q4ECJkR",
"price": "$37.00",
"extracted_price": 37,
"rating": 4.7,
"reviews": 84610,
"delivery": "$35.00 delivery",
"extensions": [
"4K Capable",
"White"
],
"durability": "Used",
"thumbnail": "https://encrypted-tbn1.gstatic.com/shopping?q=tbn:ANd9GcSpJ28gKmagcDhB_AS_UZktF0gk-iTYksqQSG57Ifxz1dlptSiqwAxKvDOrTRfr9rpTIShy2Vrz4s2D8Gn7Q6bSohuJKQhxV0Y5hk0Ja1B23DvBOM06nlNgOQ&usqp=CAE"
}
...
],
"related_results": [
{
"position": 1,
"product_id": "2085922572895778480",
"title": "PS5/PS4 - God of War Ragnarok Collector's Edition",
"link": "https://retrogamingofdenver.com/products/god-of-war-ragnarok-collectors-edition-playstation-5-new?variant=43815537017073¤cy=USD&utm_medium=product_sync&utm_source=google&utm_content=sag_organic&utm_campaign=sag_organic&srsltid=ASuE1wSenCNJ90RSI39L9OT1Fb1ZGLJL7sffKcEl8QRyU-EmbG-fVLNDYSM&rct=j&q=&esrc=s&sa=U&ved=0ahUKEwiOo_--7_f_AhWYLUQIHdFuABYQguUECJAZ&usg=AOvVaw0AxnPaaRUDjNiYlQG9ZZMX",
"product_link": "https://www.google.com/shopping/product/2085922572895778480?q=PS5&ie=UTF-8&uule=w+CAIQICIYQ2FsaWZvcm5pYSxVbml0ZWQgU3RhdGVz&oq=PS5&gl=us&hl=en&prds=pid:2502665397428699522,rsk:PC_6528732777392445047&sa=X&ved=0ahUKEwiOo_--7_f_AhWYLUQIHdFuABYQ8gIIihkoAA",
"source": "Retro Gaming of Denver",
"discount": "Apply GOOGLE$5OFF",
"price": "$169.99",
"extracted_price": 169.99,
"rating": 4.9,
"reviews": 4472,
"tag": "$5 OFF",
"delivery": "Delivery by Mon, Jul 17",
"thumbnail": "https://encrypted-tbn1.gstatic.com/shopping?q=tbn:ANd9GcTo4AdcLGHku3_Uky04DtqpeEsAW0yFzw6uSBXOEhK4BIbzpF4J32M6TPer3gx2Lpj49XGbjHPLLoRMeLVvX1VwbpEHSkVJXopTfWBwqBQRdihJXwwkhUkM&usqp=CAE"
},
{
"position": 2,
"product_id": "5395677433014263836",
"title": "Among US - Impostor Edition - PlayStation 5",
"link": "https://www.ebay.com/itm/266266230370?chn=ps&mkevt=1&mkcid=28&srsltid=ASuE1wRwrOmYXmujbiu333n9BmkaP324XHHYV5C0V9DFoCmB-7FwBxZ8jRg&rct=j&q=&esrc=s&sa=U&ved=0ahUKEwiOo_--7_f_AhWYLUQIHdFuABYQguUECKEZ&usg=AOvVaw0tZfmYMWAKXwPHygv4JWH9",
"product_link": "https://www.google.com/shopping/product/5395677433014263836?q=PS5&ie=UTF-8&uule=w+CAIQICIYQ2FsaWZvcm5pYSxVbml0ZWQgU3RhdGVz&oq=PS5&gl=us&hl=en&prds=pid:8024874914570755010,rsk:PC_13556248843592479408&sa=X&ved=0ahUKEwiOo_--7_f_AhWYLUQIHdFuABYQ8gIImxkoAA",
"source": "eBay - blackjackbones",
"price": "$14.99",
"extracted_price": 14.99,
"original_price": "Was $19.99",
"extracted_original_price": 19.99,
"rating": 4.5,
"reviews": 153,
"tag": "SALE",
"delivery": "Delivery by Thu, Jul 13",
"thumbnail": "https://encrypted-tbn3.gstatic.com/shopping?q=tbn:ANd9GcRrURHiP-Kf_BPgBZrzBUXaVwJnK0GuJTHu0RHqrzWCrPLYQUe4jWsVewYRunWhPOXOCYFj_RsX0yiR3YPGHSrHyJg838OQDTJqAJNfCfPlJLuLuN3fzOXIYQ&usqp=CAE"
},
{
"position": 3,
"product_id": "7416062647395280475",
"title": "Among Us, Crewmate Edition - PlayStation 5",
"link": "#&rct=j&q=&esrc=s&sa=U&ved=0ahUKEwiOo_--7_f_AhWYLUQIHdFuABYQguUECLMZ&usg=AOvVaw3Fr-lfi2zR_kPjnk8bGdX6",
"product_link": "https://www.google.com/shopping/product/7416062647395280475?q=PS5&ie=UTF-8&uule=w+CAIQICIYQ2FsaWZvcm5pYSxVbml0ZWQgU3RhdGVz&oq=PS5&gl=us&hl=en&prds=pid:15810759107001688362,rsk:PC_16050490641271646149&sa=X&ved=0ahUKEwiOo_--7_f_AhWYLUQIHdFuABYQ8gIIrhkoAA",
"source": "Ami Ventures",
"discount": "Discount shown at checkout",
"price": "$16.14",
"extracted_price": 16.14,
"rating": 4.4,
"reviews": 224,
"tag": "5% OFF",
"delivery": "Google Guarantee",
"thumbnail": "https://encrypted-tbn3.gstatic.com/shopping?q=tbn:ANd9GcS9pTGcSXuVhuj438UihZRTT5q6fyj65JfDL9EQjfEkNRRMaHorLqyHQnwsfj3wvOnpclfCqU6dznZYpuRAm_6Op3oowA_Bmo-oyCaNwry3-HtQKWyd1HIF&usqp=CAE"
},
{
"position": 4,
"product_id": "14739812013792815258",
"title": "Dolmen - PlayStation 5",
"link": "https://www.deepdiscount.com/goog/810086920037&rct=j&q=&esrc=s&sa=U&ved=0ahUKEwiOo_--7_f_AhWYLUQIHdFuABYQguUECMUZ&usg=AOvVaw2oB-lbnQ3CvBFGpuoLn2-V",
"product_link": "https://www.google.com/shopping/product/14739812013792815258?q=PS5&ie=UTF-8&uule=w+CAIQICIYQ2FsaWZvcm5pYSxVbml0ZWQgU3RhdGVz&oq=PS5&gl=us&hl=en&prds=pid:1765706353169471088,rsk:CID_14739812013792815258&sa=X&ved=0ahUKEwiOo_--7_f_AhWYLUQIHdFuABYQ8gIIvxkoAA",
"source": "DeepDiscount",
"price": "$19.99",
"extracted_price": 19.99,
"original_price": "Was $29.91",
"extracted_original_price": 29.91,
"rating": 3.8,
"reviews": 8,
"tag": "SALE",
"delivery": "Google Guarantee",
"thumbnail": "https://encrypted-tbn0.gstatic.com/shopping?q=tbn:ANd9GcS714yXYtxsDVEFOxZjlvxo1HV8vC7pqMK3IAgsA0ESWzfegEaMkuvvvQGMhz-RlOtVko0nHlQtFmucl_yZVCuJxIpCj-jb_9DRTGPrHMwL&usqp=CAE"
},
{
"position": 5,
"product_id": "7007321996971862281",
"title": "PS5 Rustler",
"link": "https://www.deepdiscount.com/goog/814290017088&rct=j&q=&esrc=s&sa=U&ved=0ahUKEwiOo_--7_f_AhWYLUQIHdFuABYQguUECNYZ&usg=AOvVaw14tAHkZpM_1oEwZQN06Q06",
"product_link": "https://www.google.com/shopping/product/7007321996971862281?q=PS5&ie=UTF-8&uule=w+CAIQICIYQ2FsaWZvcm5pYSxVbml0ZWQgU3RhdGVz&oq=PS5&gl=us&hl=en&prds=pid:5207733865824988147,rsk:PC_3634866181820049462&sa=X&ved=0ahUKEwiOo_--7_f_AhWYLUQIHdFuABYQ8gII0BkoAA",
"source": "DeepDiscount",
"price": "$18.98",
"extracted_price": 18.98,
"original_price": "Was $28.33",
"extracted_original_price": 28.33,
"rating": 3.6,
"reviews": 8,
"tag": "SALE",
"delivery": "Google Guarantee",
"thumbnail": "https://encrypted-tbn2.gstatic.com/shopping?q=tbn:ANd9GcQ6UVYn5Kt1l4eMpUTb4038RZCV0Hy_5T5OHSveYEcEoj9wmo8de1DvpZ9OOVMw-tOc8u-jqwk65ofm-R-MOBFwcGa8szI0zshCr-AR1jpf-RfyJCGcY9v7yg&usqp=CAE"
}
...
],
"categories": [
{
"type": "Filter by color",
"options": [
{
"title": "White",
"link": "https://www.google.com/search?gl=us&hl=en&tbm=shop&q=PS5&tbs=mr:1,pdtr0:1717142%7C1717158&sa=X&ved=0ahUKEwiOo_--7_f_AhWYLUQIHdFuABYQwQkI-BooAA",
"thumbnail": "https://encrypted-tbn3.gstatic.com/shopping?q=tbn:ANd9GcRWckHMa2moFtOE2wnS88SqFkKi0TkdGfGIdsCDsuVAClcUlYI4k2C6gfzw1OcqS2_OuNwcooalrq9pi_AODiyaycETZyJy1UiKK_4ZUxye-Onn_QTPss9E6Q&usqp=CAE"
},
{
"title": "Black",
"link": "https://www.google.com/search?gl=us&hl=en&tbm=shop&q=PS5&tbs=mr:1,pdtr0:1717142%7C1717146&sa=X&ved=0ahUKEwiOo_--7_f_AhWYLUQIHdFuABYQwQkI-xooAA",
"thumbnail": "https://encrypted-tbn0.gstatic.com/shopping?q=tbn:ANd9GcRLseD2HM2IwIWUJxHwCzE4EeokxY58wpUBAPKOE8juNo0ohaweNw-EKcDOoSEMl7s6du0aOEeh3TUADIg-hlxzB3pXGfmbzoktMoArmzr4oINdkw8wrZG4dQ&usqp=CAE"
},
{
"title": "Purple",
"link": "https://www.google.com/search?gl=us&hl=en&tbm=shop&q=PS5&tbs=mr:1,pdtr0:1717142%7C3564777&sa=X&ved=0ahUKEwiOo_--7_f_AhWYLUQIHdFuABYQwQkI_hooAA",
"thumbnail": "https://encrypted-tbn1.gstatic.com/shopping?q=tbn:ANd9GcSMHzj9ID8r8FwMywVhbHAyjAKVA41Vp5yciPI8i82ULH3HP5R46f4U-2h0S8Edt8JEmQOdudvDJkUU_DYjQTTb9HYUBj0_ucgCl791BVDWzk1zC7Bxz3An&usqp=CAE"
},
{
"title": "Red",
"link": "https://www.google.com/search?gl=us&hl=en&tbm=shop&q=PS5&tbs=mr:1,pdtr0:1717142%7C1717155&sa=X&ved=0ahUKEwiOo_--7_f_AhWYLUQIHdFuABYQwQkIgRsoAA",
"thumbnail": "https://encrypted-tbn2.gstatic.com/shopping?q=tbn:ANd9GcTTCauNY1AUDtjrPefvua2AJrdFwAvbRGg2Ygc4EqcXl0jZngw8SFy5xq353ji1njN6zZdXZiVbz9y2RQkpGfPuDkS8I5CBBpRDvb7nIRV125u6s0f7ZoOJ&usqp=CAE"
},
{
"title": "Blue",
"link": "https://www.google.com/search?gl=us&hl=en&tbm=shop&q=PS5&tbs=mr:1,pdtr0:1717142%7C1717147&sa=X&ved=0ahUKEwiOo_--7_f_AhWYLUQIHdFuABYQwQkIhBsoAA",
"thumbnail": "https://encrypted-tbn2.gstatic.com/shopping?q=tbn:ANd9GcRPDZTx2YmoQ5QWTjRxbzaor2buqEkjnGcZm6CNT401PbQRKPAMmaKVQSwi9yPs0qA26UUOVetUzttTxfnef_m4A3WoPF9p2oEalxyovpEf&usqp=CAE"
},
{
"title": "Midnight Black",
"link": "https://www.google.com/search?gl=us&hl=en&tbm=shop&q=PS5&tbs=mr:1,pdtr0:1717142%7C2188558&sa=X&ved=0ahUKEwiOo_--7_f_AhWYLUQIHdFuABYQwQkIhxsoAA",
"thumbnail": "https://encrypted-tbn0.gstatic.com/shopping?q=tbn:ANd9GcR7QX_abNpvcaHH3OZbGyDAJiUPNKLql1s9yaWLrPc3PJphWTu5salxsXOI8d7C7sJvyra6TUMIkG1ToPxDqiFWihpoAjuYHDNmETOW2aCm&usqp=CAE"
},
{
"title": "Glacier White",
"link": "https://www.google.com/search?gl=us&hl=en&tbm=shop&q=PS5&tbs=mr:1,pdtr0:1717142%7C1729539&sa=X&ved=0ahUKEwiOo_--7_f_AhWYLUQIHdFuABYQwQkIihsoAA",
"thumbnail": "https://encrypted-tbn3.gstatic.com/shopping?q=tbn:ANd9GcRkj5lw7YzxLaAxwYR0mxIjIc--dzJPIWXB9hMa351Hf4A1nQwTfR8K4djfqmqYfvImiTonsTHOZul9PTLYK0a1ku5VIIfj5FnAad1O1SbL-3NI0ktWKn2-&usqp=CAE"
}
]
},
{
"type": "Filter by wireless connectivity",
"options": [
{
"title": "Wi-Fi",
"link": "https://www.google.com/search?gl=us&hl=en&tbm=shop&q=PS5&tbs=mr:1,pdtr0:3197703%7C3197705&sa=X&ved=0ahUKEwiOo_--7_f_AhWYLUQIHdFuABYQwQkIkRsoAA",
"thumbnail": "https://encrypted-tbn3.gstatic.com/shopping?q=tbn:ANd9GcTv_9tkGYBONclSZC0KRoHdvCXffG4pC5AM187pnprv9ldqCPgeJOXciq3n5zka0owyvvnpfcSNtIVxO5nW6h7-LrOrZxtKStKzZyKKDLrVq5XyQE5JfLYGnQ&usqp=CAE"
},
{
"title": "Bluetooth",
"link": "https://www.google.com/search?gl=us&hl=en&tbm=shop&q=PS5&tbs=mr:1,pdtr0:3197703%7C3197704&sa=X&ved=0ahUKEwiOo_--7_f_AhWYLUQIHdFuABYQwQkIlBsoAA",
"thumbnail": "https://encrypted-tbn2.gstatic.com/shopping?q=tbn:ANd9GcRg-uO55MUtgUT_zlZcM24fpS9r7HXrn0HqR8kbB5SGME-yIEwo7IX8W_LZrbHRrK-9eZ6SF6tifyeAhZ5pxBQ2Jh46xLf2YMeF_UHoxceLrjRd37aVFMRRng&usqp=CAE"
}
]
}
]
}
Featured Results
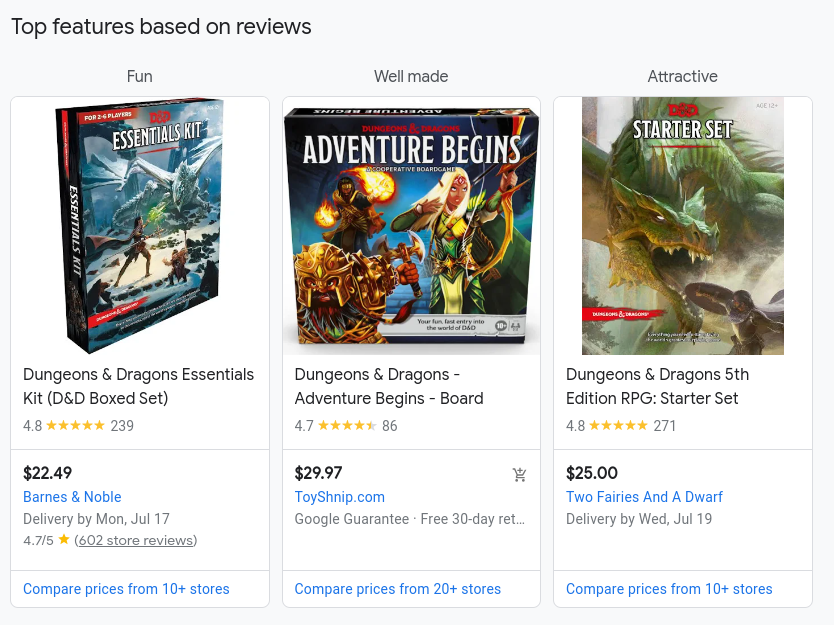
https://www.searchapi.io/api/v1/search?engine=google_shopping&location=Washington%2CUnited+States&q=Dungeons+and+Dragons
- Python
- Node
- Ruby
- Java
- Go
- PHP
- Bash
- R
- Kotlin
- Swift
- C#
- C
- C++
- requests
import requests
url = "https://www.searchapi.io/api/v1/search"
params = {
"engine": "google_shopping",
"q": "Dungeons and Dragons",
"location": "Washington,United States"
}
response = requests.get(url, params = params)
print(response.text)
{
"featured_results": [
{
"position": 1,
"product_id": "15853614032827396200",
"title": "Dungeons & Dragons Essentials Kit (D&D Boxed Set)",
"link": "https://www.barnesandnoble.com/w/dungeons-dragons-essentials-kit-wizards-rpg-team/1132716122?ean=9780786966837&rct=j&q=&esrc=s&sa=U&ved=0ahUKEwj7pO3P8Pf_AhWEKEQIHf5QDxsQguUECLAS&usg=AOvVaw3ZS6viBQbZSyGxLmN0VUjm",
"product_link": "https://www.google.com/shopping/product/15853614032827396200?q=Dungeons+and+Dragons&hl=en&uule=w+CAIQICIYV2FzaGluZ3RvbixVbml0ZWQgU3RhdGVz&gl=us&prds=eto:12038621688097915604_0,pid:4237476878884092423,rsk:PC_5928450669913291657&sa=X&ved=0ahUKEwj7pO3P8Pf_AhWEKEQIHf5QDxsQ8gIIqhIoAA",
"source": "Barnes & Noble",
"comparisons": "Compare prices from 10+ stores",
"comparisons_link": "https://www.google.com/shopping/product/15853614032827396200/offers?q=Dungeons+and+Dragons&hl=en&uule=w+CAIQICIYV2FzaGluZ3RvbixVbml0ZWQgU3RhdGVz&gl=us&prds=eto:12038621688097915604_0,pid:4237476878884092423,rsk:PC_5928450669913291657&sa=X&ved=0ahUKEwj7pO3P8Pf_AhWEKEQIHf5QDxsQ3q4ECLUS",
"price": "$22.49",
"extracted_price": 22.49,
"rating": 4.8,
"reviews": 239,
"delivery": "Delivery by Mon, Jul 17",
"remark": "Fun",
"merchant": {
"rating": 4.7,
"reviews": 602,
"link": "https://www.google.com/shopping/ratings/account/metrics?q=barnesandnoble.com&c=US&v=19&hl=en&rct=j&q=&esrc=s&sa=U&ved=0ahUKEwj7pO3P8Pf_AhWEKEQIHf5QDxsQ9-wCCLQS&usg=AOvVaw24O-KciZGNWNChn09TXGfV"
},
"thumbnail": "https://encrypted-tbn2.gstatic.com/shopping?q=tbn:ANd9GcSRCio0bAzCD93mz0FbRXPbGeVHX81CrFQnlp5uv7viCuXigV4a42X9t4se9dSDu_8vB9TKxtjzdYFP5HXpYPv4cm3bYvH6drz01dK5Fpfz_rLCkEVgqpj99g&usqp=CAE"
},
{
"position": 2,
"product_id": "17128350959726186274",
"title": "Dungeons & Dragons - Adventure Begins - Board Game",
"link": "/shopping/product/17128350959726186274?q=Dungeons+and+Dragons&hl=en&uule=w+CAIQICIYV2FzaGluZ3RvbixVbml0ZWQgU3RhdGVz&gl=us&prds=eto:13604482394156290756_1,pid:8345132313561376636,rsk:PC_7036727842417588766&rct=j&q=&esrc=s&sa=U&ved=0ahUKEwj7pO3P8Pf_AhWEKEQIHf5QDxsQguUECMIS&usg=AOvVaw3b39arrYJdpDPMwQQrCadO",
"product_link": "https://www.google.com/shopping/product/17128350959726186274?q=Dungeons+and+Dragons&hl=en&uule=w+CAIQICIYV2FzaGluZ3RvbixVbml0ZWQgU3RhdGVz&gl=us&prds=eto:13604482394156290756_1,pid:8345132313561376636,rsk:PC_7036727842417588766&sa=X&ved=0ahUKEwj7pO3P8Pf_AhWEKEQIHf5QDxsQ8gIIvBIoAA",
"source": "ToyShnip.com",
"comparisons": "Compare prices from 20+ stores",
"comparisons_link": "https://www.google.com/shopping/product/17128350959726186274/offers?q=Dungeons+and+Dragons&hl=en&uule=w+CAIQICIYV2FzaGluZ3RvbixVbml0ZWQgU3RhdGVz&gl=us&prds=eto:13604482394156290756_1,pid:8345132313561376636,rsk:PC_7036727842417588766&sa=X&ved=0ahUKEwj7pO3P8Pf_AhWEKEQIHf5QDxsQ3q4ECMUS",
"price": "$29.97",
"extracted_price": 29.97,
"rating": 4.7,
"reviews": 86,
"delivery": "Google Guarantee · Free 30-day returns",
"remark": "Well made",
"thumbnail": "https://encrypted-tbn2.gstatic.com/shopping?q=tbn:ANd9GcSe9t7cNuwQpPn3OUjqnUgwC_wNLGEpsEE5gOweS4v2DAusgsLlrEnhf1_EpNWilOGEiSquz5H0tiFQYYwZW_c21w79YXIa1exfqKlCe6m2ifLHDWNNITiN3g&usqp=CAE"
},
{
"position": 3,
"product_id": "13057181074707634237",
"title": "Dungeons & Dragons 5th Edition RPG: Starter Set",
"link": "https://twofairiesandadwarf.com/products/beginner-box-d-d-5nd-edition?variant=40128442040471¤cy=USD&utm_medium=product_sync&utm_source=google&utm_content=sag_organic&utm_campaign=sag_organic&srsltid=ASuE1wQjBXuf9MZDs5-ZCng3vS_k1XrK4u-z08rdZIkLJS9lgcx4rzxQ2M4&rct=j&q=&esrc=s&sa=U&ved=0ahUKEwj7pO3P8Pf_AhWEKEQIHf5QDxsQguUECNIS&usg=AOvVaw04xPYZPVEk_E5guriPKsa9",
"product_link": "https://www.google.com/shopping/product/13057181074707634237?q=Dungeons+and+Dragons&hl=en&uule=w+CAIQICIYV2FzaGluZ3RvbixVbml0ZWQgU3RhdGVz&gl=us&prds=eto:14528264265873608038_0,pid:3381827932805029950,rsk:PC_12669569499330260161&sa=X&ved=0ahUKEwj7pO3P8Pf_AhWEKEQIHf5QDxsQ8gIIzBIoAA",
"source": "Two Fairies And A Dwarf",
"comparisons": "Compare prices from 10+ stores",
"comparisons_link": "https://www.google.com/shopping/product/13057181074707634237/offers?q=Dungeons+and+Dragons&hl=en&uule=w+CAIQICIYV2FzaGluZ3RvbixVbml0ZWQgU3RhdGVz&gl=us&prds=eto:14528264265873608038_0,pid:3381827932805029950,rsk:PC_12669569499330260161&sa=X&ved=0ahUKEwj7pO3P8Pf_AhWEKEQIHf5QDxsQ3q4ECNUS",
"price": "$25.00",
"extracted_price": 25,
"rating": 4.8,
"reviews": 271,
"delivery": "Delivery by Wed, Jul 19",
"remark": "Attractive",
"thumbnail": "https://encrypted-tbn2.gstatic.com/shopping?q=tbn:ANd9GcScvteVI7rnnXQk7X9Z-AVKR3ijxqYWdnWL-hP4DW1pYIsENPTex7mOhWTP24gjMEwCC_0hOrA7njl7usJBHOAMyAR13xXVDOMFzS4lSgxg&usqp=CAE"
}
]
}
Shopping Results
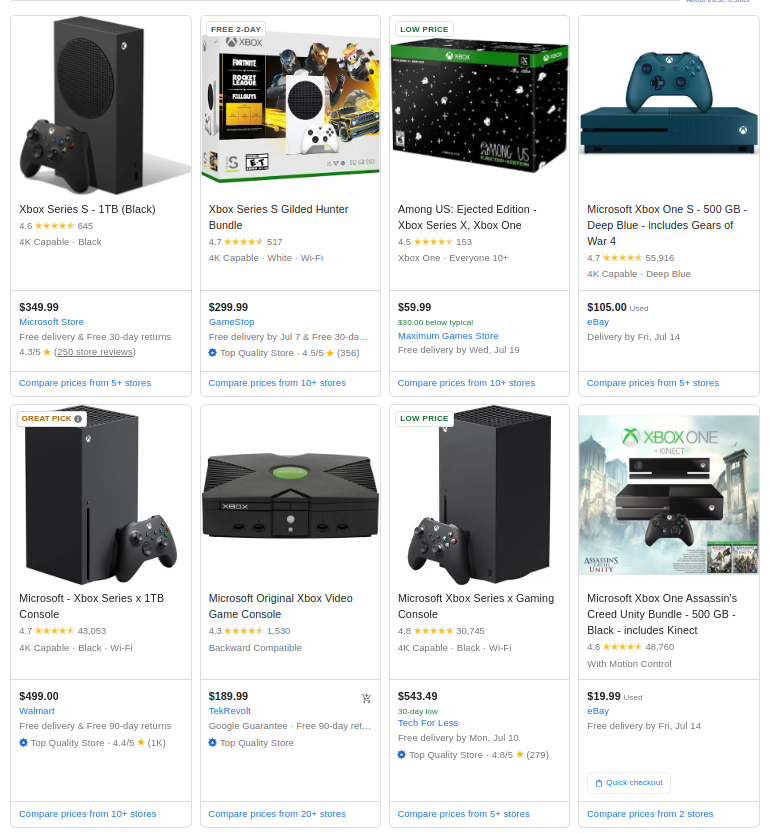
https://www.searchapi.io/api/v1/search?engine=google_shopping&location=Miami-Dade+County%2CFlorida%2CUnited+States&q=XBOX
- Python
- Node
- Ruby
- Java
- Go
- PHP
- Bash
- R
- Kotlin
- Swift
- C#
- C
- C++
- requests
import requests
url = "https://www.searchapi.io/api/v1/search"
params = {
"engine": "google_shopping",
"q": "XBOX",
"location": "Miami-Dade County,Florida,United States"
}
response = requests.get(url, params = params)
print(response.text)
{
"shopping_results": [
{
"position": 1,
"product_id": "18406579019672098267",
"title": "Xbox Series S - 1TB (Black)",
"link": "https://www.microsoft.com/en-us/d/xbox-series-s--1tb-black/8ZCBGTT29H9C/DMXV?OCID=cmm6mu07qw1_seo_omc_goo&source=googleshopping&rct=j&q=&esrc=s&sa=U&ved=0ahUKEwiK7Ly08ff_AhWEGIgKHS_KDzUQguUECI0O&usg=AOvVaw15RKLfmybR8O7MysZ5Qena",
"product_link": "https://www.google.com/shopping/product/18406579019672098267?uule=w+CAIQICInTWlhbWktRGFkZSBDb3VudHksRmxvcmlkYSxVbml0ZWQgU3RhdGVz&hl=en&oq=XBOX&gl=us&q=XBOX&prds=eto:5146653718602334900_0,pid:3217562886526249831,rsk:PC_6566645158571678763&sa=X&ved=0ahUKEwiK7Ly08ff_AhWEGIgKHS_KDzUQ8gIIhQ4oAA",
"source": "Microsoft Store",
"comparisons": "Compare prices from 5+ stores",
"comparisons_link": "https://www.google.com/shopping/product/18406579019672098267/offers?uule=w+CAIQICInTWlhbWktRGFkZSBDb3VudHksRmxvcmlkYSxVbml0ZWQgU3RhdGVz&hl=en&oq=XBOX&gl=us&q=XBOX&prds=eto:5146653718602334900_0,pid:3217562886526249831,rsk:PC_6566645158571678763&sa=X&ved=0ahUKEwiK7Ly08ff_AhWEGIgKHS_KDzUQ3q4ECJIO",
"price": "$349.99",
"extracted_price": 349.99,
"rating": 4.6,
"reviews": 645,
"delivery": "Free delivery & Free 30-day returns",
"extensions": [
"4K Capable",
"Black"
],
"merchant": {
"rating": 4.3,
"reviews": 55,
"link": "https://www.google.com/shopping/ratings/account/metrics?q=microsoft.com&c=US&v=19&hl=en&rct=j&q=&esrc=s&sa=U&ved=0ahUKEwiK7Ly08ff_AhWEGIgKHS_KDzUQ9-wCCJEO&usg=AOvVaw0p6LO7ZbVkgrJB6lFkpnZ2"
},
"thumbnail": "https://encrypted-tbn1.gstatic.com/shopping?q=tbn:ANd9GcRlPE-sDmtBWoK3jJZYX-CJobaydHaJzlm8Mop1ZE2hgUV99QO7V4STB4wyKvVBKF35014JI19Drmxkg4V_4zb3X1z8MlpHEAkyeVLQj0yfOn1zoCKmZ-jg&usqp=CAE"
},
{
"position": 2,
"product_id": "10315764599879528351",
"title": "Xbox Series S Gilded Hunter Bundle",
"link": "https://www.gamestop.com/consoles-hardware/xbox-series-x%7Cs/consoles/products/microsoft-xbox-series-s-digital-edition-console---gilded-hunter-bundle/352659.html&rct=j&q=&esrc=s&sa=U&ved=0ahUKEwiK7Ly08ff_AhWEGIgKHS_KDzUQguUECKIO&usg=AOvVaw2eEnXWLrXNFfgdI7sh15jr",
"product_link": "https://www.google.com/shopping/product/10315764599879528351?uule=w+CAIQICInTWlhbWktRGFkZSBDb3VudHksRmxvcmlkYSxVbml0ZWQgU3RhdGVz&hl=en&oq=XBOX&gl=us&q=XBOX&prds=eto:4100800704414278612_0,pid:8927120433859451976,rsk:PC_10103495928327981560&sa=X&ved=0ahUKEwiK7Ly08ff_AhWEGIgKHS_KDzUQ8gIImg4oAA",
"source": "GameStop",
"comparisons": "Compare prices from 10+ stores",
"comparisons_link": "https://www.google.com/shopping/product/10315764599879528351/offers?uule=w+CAIQICInTWlhbWktRGFkZSBDb3VudHksRmxvcmlkYSxVbml0ZWQgU3RhdGVz&hl=en&oq=XBOX&gl=us&q=XBOX&prds=eto:4100800704414278612_0,pid:8927120433859451976,rsk:PC_10103495928327981560&sa=X&ved=0ahUKEwiK7Ly08ff_AhWEGIgKHS_KDzUQ3q4ECKoO",
"price": "$299.99",
"extracted_price": 299.99,
"rating": 4.7,
"reviews": 517,
"tag": "FREE 2-DAY",
"delivery": "Free delivery by Jul 7 & Free 30-day returns",
"badge": "Top Quality Store",
"extensions": [
"4K Capable",
"White",
"Wi-Fi"
],
"merchant": {
"rating": 4.5,
"reviews": 356,
"link": "https://www.google.com/shopping/ratings/account/metrics?q=gamestop.com&c=US&v=19&hl=en&rct=j&q=&esrc=s&sa=U&ved=0ahUKEwiK7Ly08ff_AhWEGIgKHS_KDzUQ9-wCCKkO&usg=AOvVaw3it7euYIq1msG0sBisHFtw"
},
"thumbnail": "https://encrypted-tbn2.gstatic.com/shopping?q=tbn:ANd9GcQEz9gNMJa5iTK7E6f0GK8dg7ze0XMEEweZIjGPJjtdwfGLmxysRKayz8mAHofAAqBOITlxgoKwHTkuTJVXP8k3ak7MpzVH9l9-yedzlTM0jaDaKGzo9bni_w&usqp=CAE"
},
{
"position": 3,
"product_id": "2426654963215995323",
"title": "Among US: Ejected Edition - Xbox Series X, Xbox One",
"link": "https://store.maximumgames.com/products/among-us-ejected-edition?variant=39391368249459¤cy=USD&utm_medium=product_sync&utm_source=google&utm_content=sag_organic&utm_campaign=sag_organic&rct=j&q=&esrc=s&sa=U&ved=0ahUKEwiK7Ly08ff_AhWEGIgKHS_KDzUQguUECLoO&usg=AOvVaw2c1qVT2tgEUooBfVPa0d8r",
"product_link": "https://www.google.com/shopping/product/2426654963215995323?uule=w+CAIQICInTWlhbWktRGFkZSBDb3VudHksRmxvcmlkYSxVbml0ZWQgU3RhdGVz&hl=en&oq=XBOX&gl=us&q=XBOX&prds=eto:28018886890524680_0,pid:577702996197606981,rsk:PC_13556248843592479408&sa=X&ved=0ahUKEwiK7Ly08ff_AhWEGIgKHS_KDzUQ8gIIsg4oAA",
"source": "Maximum Games Store",
"comparisons": "Compare prices from 10+ stores",
"comparisons_link": "https://www.google.com/shopping/product/2426654963215995323/offers?uule=w+CAIQICInTWlhbWktRGFkZSBDb3VudHksRmxvcmlkYSxVbml0ZWQgU3RhdGVz&hl=en&oq=XBOX&gl=us&q=XBOX&prds=eto:28018886890524680_0,pid:577702996197606981,rsk:PC_13556248843592479408&sa=X&ved=0ahUKEwiK7Ly08ff_AhWEGIgKHS_KDzUQ3q4ECL4O",
"price": "$59.99",
"extracted_price": 59.99,
"rating": 4.5,
"reviews": 153,
"tag": "LOW PRICE",
"delivery": "Free delivery by Wed, Jul 19",
"extensions": [
"Xbox One",
"Everyone 10+"
],
"thumbnail": "https://encrypted-tbn3.gstatic.com/shopping?q=tbn:ANd9GcRoE4KM7Oykt7P5-afirkG2zwzx3UCPBReEGwPVYlUNNrYlqnj6qw_qBCYO-gTWG_5ZZnH-_leIjCtaDYYs3aWCGttViuGhOZKwlnl68AfmILJKz3tEzAUF&usqp=CAE"
},
{
"position": 4,
"product_id": "15229711540759715443",
"title": "Microsoft Xbox One S - 500 GB - Deep Blue - includes Gears of War 4",
"link": "https://www.ebay.com/itm/225617134062?chn=ps&mkevt=1&mkcid=28&srsltid=ASuE1wQkJRT-ylBzb_EaHt3CYWfkbCYb_tRkk3m-PTfYBZhIzppTFGsUxt0&rct=j&q=&esrc=s&sa=U&ved=0ahUKEwiK7Ly08ff_AhWEGIgKHS_KDzUQguUECMwO&usg=AOvVaw0zpKfWkDLnTA_3sz9DPoos",
"product_link": "https://www.google.com/shopping/product/15229711540759715443?uule=w+CAIQICInTWlhbWktRGFkZSBDb3VudHksRmxvcmlkYSxVbml0ZWQgU3RhdGVz&hl=en&oq=XBOX&gl=us&q=XBOX&prds=eto:5260777486245653426_0,pid:16500744374613252998,rsk:PC_8872961111726356845&sa=X&ved=0ahUKEwiK7Ly08ff_AhWEGIgKHS_KDzUQ8gIIxA4oAA",
"source": "eBay",
"comparisons": "Compare prices from 5+ stores",
"comparisons_link": "https://www.google.com/shopping/product/15229711540759715443/offers?uule=w+CAIQICInTWlhbWktRGFkZSBDb3VudHksRmxvcmlkYSxVbml0ZWQgU3RhdGVz&hl=en&oq=XBOX&gl=us&q=XBOX&prds=eto:5260777486245653426_0,pid:16500744374613252998,rsk:PC_8872961111726356845&sa=X&ved=0ahUKEwiK7Ly08ff_AhWEGIgKHS_KDzUQ3q4ECNAO",
"price": "$105.00",
"extracted_price": 105,
"rating": 4.7,
"reviews": 55916,
"delivery": "Delivery by Fri, Jul 14",
"extensions": [
"4K Capable",
"Deep Blue"
],
"durability": "Used",
"thumbnail": "https://encrypted-tbn0.gstatic.com/shopping?q=tbn:ANd9GcQFWP-QPLtLQkQONml5c0RzBZ08ZioHDNL1ptr1qSyC742yG2vUlU620Pam641tXvwvDi9IYYbI5ZSlUVg97dj9WtfttCulDEG28KLp7QmHrX2sNJV2dUG3&usqp=CAE"
},
{
"position": 5,
"product_id": "18645849156115410",
"title": "Microsoft - Xbox Series x 1TB Console",
"link": "https://www.walmart.com/ip/Xbox-Series-X-Video-Game-Console-Black/443574645?wmlspartner=wlpa&selectedSellerId=0&rct=j&q=&esrc=s&sa=U&ved=0ahUKEwiK7Ly08ff_AhWEGIgKHS_KDzUQguUECOEO&usg=AOvVaw2gEpi4OMWC8gq48tEEKATg",
"product_link": "https://www.google.com/shopping/product/18645849156115410?uule=w+CAIQICInTWlhbWktRGFkZSBDb3VudHksRmxvcmlkYSxVbml0ZWQgU3RhdGVz&hl=en&oq=XBOX&gl=us&q=XBOX&prds=eto:13486746012170777061_0,pid:9738872028475260018,rsk:PC_12635063620587454102&sa=X&ved=0ahUKEwiK7Ly08ff_AhWEGIgKHS_KDzUQ8gII2A4oAA",
"source": "Walmart",
"comparisons": "Compare prices from 10+ stores",
"comparisons_link": "https://www.google.com/shopping/product/18645849156115410/offers?uule=w+CAIQICInTWlhbWktRGFkZSBDb3VudHksRmxvcmlkYSxVbml0ZWQgU3RhdGVz&hl=en&oq=XBOX&gl=us&q=XBOX&prds=eto:13486746012170777061_0,pid:9738872028475260018,rsk:PC_12635063620587454102&sa=X&ved=0ahUKEwiK7Ly08ff_AhWEGIgKHS_KDzUQ3q4ECOkO",
"price": "$499.00",
"extracted_price": 499,
"rating": 4.7,
"reviews": 43053,
"tag": "GREAT PICKThis product and store are highly rated, and the price is competitive.",
"delivery": "Free delivery & Free 90-day returns",
"badge": "Top Quality Store",
"extensions": [
"4K Capable",
"Black",
"Wi-Fi"
],
"merchant": {
"rating": 4.4,
"reviews": 1000,
"link": "https://www.google.com/shopping/ratings/account/metrics?q=walmart.com&c=US&v=19&hl=en&rct=j&q=&esrc=s&sa=U&ved=0ahUKEwiK7Ly08ff_AhWEGIgKHS_KDzUQ9-wCCOgO&usg=AOvVaw2RMnVvzvluZg6hajBgqZi7"
},
"thumbnail": "https://encrypted-tbn3.gstatic.com/shopping?q=tbn:ANd9GcQqJ-BVWRVFi1GPeAJ86GVzLhgvFxzustYpdg2khfwDkr9MENf07OPOujcTlGJ3Pp2JTMQzQ_SDjH9X6w6bY1VixZq3SkaVzS9WTqHelnGE&usqp=CAE"
},
{
"position": 6,
"product_id": "14116359292397510878",
"title": "Microsoft Original Xbox Video Game Console",
"link": "http://www.google.com/url?url=/shopping/product/14116359292397510878?uule=w+CAIQICInTWlhbWktRGFkZSBDb3VudHksRmxvcmlkYSxVbml0ZWQgU3RhdGVz&hl=en&oq=XBOX&gl=us&q=XBOX&prds=eto:2511433338712182907_1,pid:13961676708257216603,rsk:PC_11482472231517888874&rct=j&q=&esrc=s&sa=U&ved=0ahUKEwiK7Ly08ff_AhWEGIgKHS_KDzUQguUECPYO&usg=AOvVaw1QcdKKVa23uv3zIHKqD6qE",
"product_link": "https://www.google.com/shopping/product/14116359292397510878?uule=w+CAIQICInTWlhbWktRGFkZSBDb3VudHksRmxvcmlkYSxVbml0ZWQgU3RhdGVz&hl=en&oq=XBOX&gl=us&q=XBOX&prds=eto:2511433338712182907_1,pid:13961676708257216603,rsk:PC_11482472231517888874&sa=X&ved=0ahUKEwiK7Ly08ff_AhWEGIgKHS_KDzUQ8gII8A4oAA",
"source": "TekRevolt",
"comparisons": "Compare prices from 20+ stores",
"comparisons_link": "https://www.google.com/shopping/product/14116359292397510878/offers?uule=w+CAIQICInTWlhbWktRGFkZSBDb3VudHksRmxvcmlkYSxVbml0ZWQgU3RhdGVz&hl=en&oq=XBOX&gl=us&q=XBOX&prds=eto:2511433338712182907_1,pid:13961676708257216603,rsk:PC_11482472231517888874&sa=X&ved=0ahUKEwiK7Ly08ff_AhWEGIgKHS_KDzUQ3q4ECPwO",
"price": "$189.99",
"extracted_price": 189.99,
"rating": 4.3,
"reviews": 1530,
"delivery": "Google Guarantee · Free 90-day returns",
"badge": "Top Quality Store",
"extensions": [
"Backward Compatible"
],
"thumbnail": "https://encrypted-tbn0.gstatic.com/shopping?q=tbn:ANd9GcQ1ZynLdXiLSlhm9EeEOyoZn1sajWqx9fYIRMAFv-ihlrwWyjONxI4LW56JTFGdADaG6gwkotA37KJ4UaQSNKgNmVa8V0gV5cW1qb-zJKIDP3T9SwuF5evc6g&usqp=CAE"
}
...
]
}
Shopping Ads
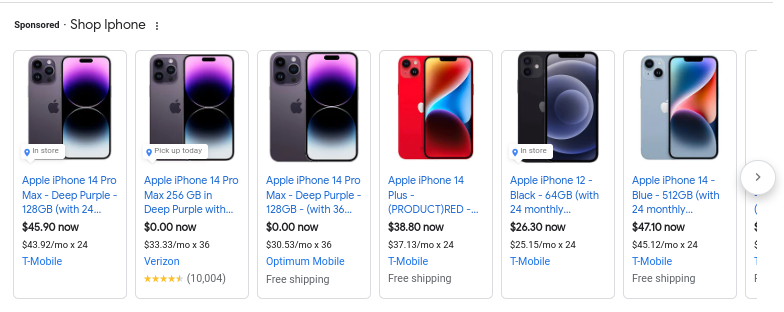
https://www.searchapi.io/api/v1/search?engine=google_shopping&location=New+York%2CUnited+States&q=Iphone
- Python
- Node
- Ruby
- Java
- Go
- PHP
- Bash
- R
- Kotlin
- Swift
- C#
- C
- C++
- requests
import requests
url = "https://www.searchapi.io/api/v1/search"
params = {
"engine": "google_shopping",
"q": "Iphone",
"location": "New York,United States"
}
response = requests.get(url, params = params)
print(response.text)
{
"shopping_ads": [
{
"position": 1,
"block_position": "top",
"title": "Apple iPhone 14 Pro Max - Deep Purple - 128GB (with 24 monthly payments)",
"link": "https://www.google.com/aclk?...",
"seller": "T-Mobile",
"price": "$45.90 now",
"extracted_price": 45.9,
"order_fullfillmed_method": "In store",
"installment": {
"down_payment": "$45.90 now",
"extracted_down_payment": 45.9,
"months": "24",
"extracted_months": 24,
"cost_per_month": "$43.92/mo",
"extracted_cost_per_moth": 43.92
},
"image": "https://encrypted-tbn2.gstatic.com/shopping?q=tbn:ANd9GcQWENlprVxy5iCaXQpSp7dFhRxYKfBCdPdsaUCP0i6ZZHTcA3HwRHrAr67aIjWAScjoGt8wmLloB3WROajIZVnezkwoqtpNx2gNZB7BxzkUFvJs4eWGYEdA&usqp=CAE"
},
{
"position": 2,
"block_position": "top",
"title": "Apple iPhone 14 Pro Max 256 GB in Deep Purple with installment",
"link": "https://www.google.com/aclk?...",
"seller": "Verizon",
"rating": 4.5,
"reviews": 10004,
"price": "$0.00 now",
"extracted_price": 0,
"order_fullfillmed_method": "Pick up today",
"installment": {
"down_payment": "$0.00 now",
"extracted_down_payment": 0,
"months": "36",
"extracted_months": 36,
"cost_per_month": "$33.33/mo",
"extracted_cost_per_moth": 33.33
},
"image": "https://encrypted-tbn1.gstatic.com/shopping?q=tbn:ANd9GcQzsBsfiSJyW2mmoz3EBUiuDarEe6iFCqnJ-80E3hkrxbXWQ9HJmjUvMJs85JQ7OjmYRyeVL3h0310XCp6GiZCk4ngeJHP8kBZCTw0q7lSW&usqp=CAE"
},
{
"position": 3,
"block_position": "top",
"title": "Apple iPhone 14 Pro Max - Deep Purple - 128GB - (with 36 monthly installment payments + plan)",
"link": "https://www.google.com/aclk?...",
"seller": "Optimum Mobile",
"price": "$0.00 now",
"extracted_price": 0,
"installment": {
"down_payment": "$0.00 now",
"extracted_down_payment": 0,
"months": "36",
"extracted_months": 36,
"cost_per_month": "$30.53/mo",
"extracted_cost_per_moth": 30.53
},
"image": "https://encrypted-tbn0.gstatic.com/shopping?q=tbn:ANd9GcSFSKoEngDP98w1nadnfw5scxhtriqR_d0T141tqYz-22S1CotUrgM5fVw-CP8GKkFyvgZ6aCXPS_RpjxnGxTS6iStz6h_lTd9WjNLwQR0n-5mHYvQTVKHb&usqp=CAE"
},
{
"position": 4,
"block_position": "top",
"title": "Apple iPhone 14 Plus - (PRODUCT)RED - 128GB (with 24 monthly payments)",
"link": "https://www.google.com/aclk?...",
"seller": "T-Mobile",
"price": "$38.80 now",
"extracted_price": 38.8,
"installment": {
"down_payment": "$38.80 now",
"extracted_down_payment": 38.8,
"months": "24",
"extracted_months": 24,
"cost_per_month": "$37.13/mo",
"extracted_cost_per_moth": 37.13
},
"image": "https://encrypted-tbn0.gstatic.com/shopping?q=tbn:ANd9GcRx7uqgaymgKYKcibXgArf62jujg3Tf6ewJFJtmcHg3DOVqRLul-P7cIURDwbjnHRaFAlUXqr0NTsmydBVIvXowhcHrmMKVvF5llBwxRXLOgT62gfumYnJmNw&usqp=CAE"
},
{
"position": 5,
"block_position": "top",
"title": "Apple iPhone 12 - Black - 64GB (with 24 monthly payments)",
"link": "https://www.google.com/aclk?...",
"seller": "T-Mobile",
"price": "$26.30 now",
"extracted_price": 26.3,
"order_fullfillmed_method": "In store",
"installment": {
"down_payment": "$26.30 now",
"extracted_down_payment": 26.3,
"months": "24",
"extracted_months": 24,
"cost_per_month": "$25.15/mo",
"extracted_cost_per_moth": 25.15
},
"image": "https://encrypted-tbn3.gstatic.com/shopping?q=tbn:ANd9GcTZsv8zJR7GpJ6FNO7e-os-_YjMdx5_caf9chuCC2nzrfF0s5mv_2Fa_CQRKdI8ysGqh6BPSP93pUp6sWiwt5opDecWw-PUOgxjsBRzKVQCJ9JzzcB9F6wr&usqp=CAE"
},
{
"position": 6,
"block_position": "top",
"title": "Apple iPhone 14 - Blue - 512GB (with 24 monthly payments)",
"link": "https://www.google.com/aclk?...",
"seller": "T-Mobile",
"price": "$47.10 now",
"extracted_price": 47.1,
"installment": {
"down_payment": "$47.10 now",
"extracted_down_payment": 47.1,
"months": "24",
"extracted_months": 24,
"cost_per_month": "$45.12/mo",
"extracted_cost_per_moth": 45.12
},
"image": "https://encrypted-tbn1.gstatic.com/shopping?q=tbn:ANd9GcT8j9wqxGD2_N7F-epE8HmDVRM4qKV3dBK4gMfW4CWTf2G8h1RZLEVuromiecUAI0Aosngp_MYDt_flQBggk6A9HinAeBxRCxTno9aYHA2esnHudX-aIc-BYQ&usqp=CAE"
}
...
]
}
Nearby Results
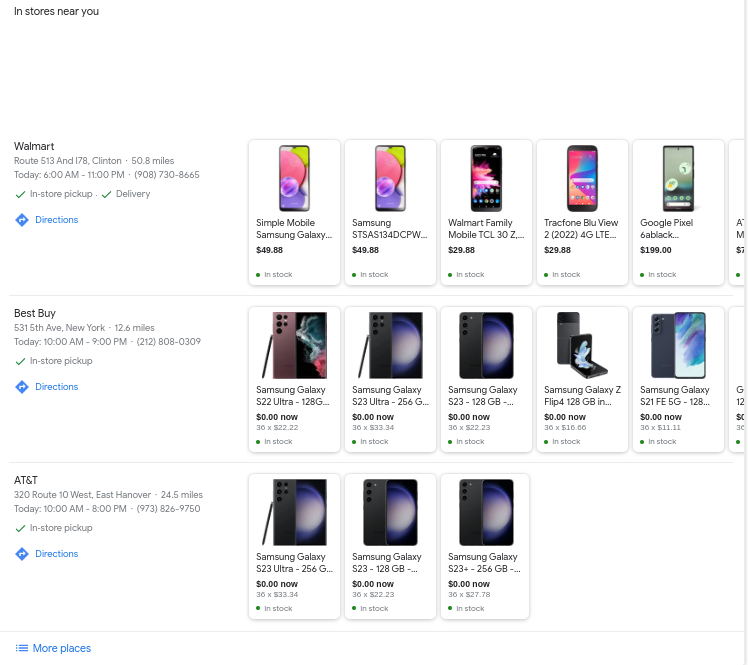
https://www.searchapi.io/api/v1/search?engine=google_shopping&location=New+York%2CUnited+States&q=Android&tbs=mr%3A1%2Clocal_avail%3A1%2Css%3A55
- Python
- Node
- Ruby
- Java
- Go
- PHP
- Bash
- R
- Kotlin
- Swift
- C#
- C
- C++
- requests
import requests
url = "https://www.searchapi.io/api/v1/search"
params = {
"engine": "google_shopping",
"q": "Android",
"location": "New York,United States",
"tbs": "mr:1,local_avail:1,ss:55"
}
response = requests.get(url, params = params)
print(response.text)
{
"nearby_results_map": {
"link": "https://www.google.com/maps/search/Android?hl=en&gl=us&sa=X&ved=0ahUKEwi3yKHT8vf_AhXcJEQIHTs8Dk8Qg4kFCIwR",
"thumbnail": "https://maps.google.com/maps/api/staticmap?key=AIzaSyA9uuHR0fCSAoZnQ1Eq4MY3WbKrv1nHDrg&maptype=roadmap&language=en®ion=US&ei=Bo6lZLetNdzJkPIPu_i4-AQ&scale=2&size=1024x136&markers=icon:http://www.gstatic.com/circulars/shared/lia_store_medium_2x.png%7Cscale:2%7C40.628486,-74.921319%7C40.754842,-73.979685%7C40.804679,-74.365902&markers=icon:http://www.gstatic.com/circulars/shared/lia_dot_small_2x.png%7Cscale:2%7C41.310664,-73.866716%7C40.224999,-74.085270%7C40.742619,-73.992714%7C40.593617,-73.999141%7C40.774032,-73.733144%7C40.674143,-73.975482%7C40.900402,-73.818564%7C40.824371,-73.836898%7C40.783766,-73.833326%7C40.737873,-73.612586%7C40.906944,-74.133265%7C40.926032,-73.855368%7C40.739561,-73.585821%7C40.456001,-74.401469%7C40.862854,-73.128835%7C40.779407,-73.954722%7C40.861798,-73.894186%7C40.863515,-73.129405%7C41.229341,-73.226774%7C41.063587,-74.128007%7C40.738371,-73.613146%7C40.692501,-73.507334%7C40.649465,-73.889416%7C40.803523,-74.362735%7C40.867161,-73.825437%7C40.706217,-73.807145%7C40.739304,-73.994090%7C40.713722,-73.759231&signature=nCA95xiIPl2Rn8z7g3veUMMrlhA%3D"
},
"nearby_results": [
{
"title": "Walmart",
"link": "https://www.google.com/search?gl=us&hl=en&q=Walmart&ludocid=1780331645050934809&gsas=1&ibp=gwp;0,7&lsig=AB86z5XLID3cFWQc-HnpepjU_C0r&sa=X&ved=0ahUKEwi3yKHT8vf_AhXcJEQIHTs8Dk8QgYkFCI4RKAA",
"address": "Route 513 And I78, Clinton",
"distance": "50.8 miles",
"working_hours": "Today: 6:00 AM - 11:00 PM",
"phone": "(908) 730-8665",
"delivery_options": [
"In‑store pickup",
"Delivery"
],
"direction": "https://maps.google.com/maps?gl=us&uule=w+CAIQICIWTmV3IFlvcmssVW5pdGVkIFN0YXRlcw&hl=en&daddr=Route+513+And+I78,+Clinton,+NJ+08809,+United+States",
"items": [
{
"title": "Simple Mobile Samsung Galaxy A03s, 32gb, Black- Prepaid Smartphone",
"link": "https://www.walmart.com/ip/Simple-Mobile-Samsung-Galaxy-A03s-32GB-Black-Prepaid-Smartphone-Locked-to-Simple-Mobile/405272661?wl13=2582&selectedSellerId=0&rct=j&q=&esrc=s&sa=U&ved=0ahUKEwi3yKHT8vf_AhXcJEQIHTs8Dk8QgokFCJMRKAA&usg=AOvVaw0I9uPh8_6HmFXSNOaubGaJ",
"price": "$49.88",
"extracted_price": 49.88,
"stock_information": "In stock",
"thumbnail": "https://encrypted-tbn3.gstatic.com/shopping?q=tbn:ANd9GcSMGI0xF4nf4nZjyyQMuv_0iiyTV5s46JCussntepuJI3xpcM3SPbHdDUOJ35sGeLxOnWk_kk_-MzT_k5qhk0qLlfdhM6ZXEm5HTPwfVKIIQivcnkC5am2Emw&usqp=CAE"
},
{
"title": "Samsung STSAS134DCPWP Straight Talk Galaxy A03s, 32gb, Black - Prepaid Smartphone",
"link": "https://www.walmart.com/ip/Straight-Talk-Samsung-Galaxy-A03s-32GB-Black-Prepaid-Smartphone-Locked-to-Straight-Talk/706770009?wl13=2582&selectedSellerId=0&rct=j&q=&esrc=s&sa=U&ved=0ahUKEwi3yKHT8vf_AhXcJEQIHTs8Dk8QgokFCJURKAA&usg=AOvVaw3fk__mEjwyBKGN2JGF7_Vf",
"price": "$49.88",
"extracted_price": 49.88,
"stock_information": "In stock",
"thumbnail": "https://encrypted-tbn1.gstatic.com/shopping?q=tbn:ANd9GcQ2oQBvmNKNn8hy5Ps052d77CYpADqMukw9823J-GlGh-9EURDg4PALTGdHD01adNtJ5nmdrS3_-MU-uPQA7jaNCZMq9Sl7rmQ9OxfT8bJpZFkLxFaOF0zGdw&usqp=CAE"
},
{
"title": "Walmart Family Mobile TCL 30 Z, 32gb, Black- Prepaid Smartphone",
"link": "https://www.walmart.com/ip/Walmart-Family-Mobile-TCL-30-Z-32GB-Black-Prepaid-Smartphone/972364678?wl13=2582&selectedSellerId=0&rct=j&q=&esrc=s&sa=U&ved=0ahUKEwi3yKHT8vf_AhXcJEQIHTs8Dk8QgokFCJcRKAA&usg=AOvVaw1awnae9Bc901pKQJsR8I5q",
"price": "$29.88",
"extracted_price": 29.88,
"stock_information": "In stock",
"thumbnail": "https://encrypted-tbn2.gstatic.com/shopping?q=tbn:ANd9GcQu42pr-eDDV8OvE-OmFawFOYLdODcYeV2-4cX_vHtXsqRkNxjkYsCmNIrsuMJY6fipahmwPZUbk575ICC5zxY9GHfzqHxJXbSHLvxUteLOE79OTG7pdF7Hgw&usqp=CAE"
},
{
"title": "Tracfone Blu View 2 (2022) 4G LTE 32gb Sim Card Included Black - Prepaid Smartphone (Locked)",
"link": "https://www.walmart.com/ip/Tracfone-BLU-View-2-32GB-Black-Prepaid-Smartphone-Locked-to-Carrier-Tracfone/105215108?wl13=2582&selectedSellerId=0&rct=j&q=&esrc=s&sa=U&ved=0ahUKEwi3yKHT8vf_AhXcJEQIHTs8Dk8QgokFCJkRKAA&usg=AOvVaw2NFGtvAyRa-b4_orr1VRlk",
"price": "$29.88",
"extracted_price": 29.88,
"stock_information": "In stock",
"thumbnail": "https://encrypted-tbn3.gstatic.com/shopping?q=tbn:ANd9GcSqTreIhaqFXYGLx2i5KuEd8-nm-8rhxNWzbgiWRRpSjIyyi224BuliVRH2tQEEyidMqJNYuKY2XhXZ6r4DjYvBecGUDB-sfCseNTWkFI8RlX_KB_Pm2sNOkw&usqp=CAE"
},
{
"title": "Google Pixel 6ablack Smartphone",
"link": "https://www.walmart.com/ip/Straight-Talk-Google-Pixel-6A-128GB-Black-Prepaid-Smartphone-Locked-to-Straight-Talk/2092871893?wl13=2582&selectedSellerId=0&rct=j&q=&esrc=s&sa=U&ved=0ahUKEwi3yKHT8vf_AhXcJEQIHTs8Dk8QgokFCJsRKAA&usg=AOvVaw3XBio-q51j54ermqxgi0Je",
"price": "$199.00",
"extracted_price": 199,
"stock_information": "In stock",
"thumbnail": "https://encrypted-tbn3.gstatic.com/shopping?q=tbn:ANd9GcSBEm1Q76Rc1U9Suk9lIfI2RFTbB0GXQjduTDrdERkU1hcnWI0ud6En-5Rhtg9uiyS9IQlPUPlkC3xkrbCxo1sKT8BhWJIGCiviiVhJtfZzcP77vXBxg5MBLQ&usqp=CAE"
},
{
"title": "AT&T Prepaid Motorola Moto G Power (64GB) - Black",
"link": "https://www.walmart.com/ip/AT-T-Motorola-Moto-g-Power-64GB-Dark-Grove-Prepaid-Smartphone/507790491?wl13=2582&selectedSellerId=0&rct=j&q=&esrc=s&sa=U&ved=0ahUKEwi3yKHT8vf_AhXcJEQIHTs8Dk8QgokFCJ0RKAA&usg=AOvVaw1Np4Fz7tvLvrlOJrkY7ILh",
"price": "$79.88",
"extracted_price": 79.88,
"stock_information": "In stock",
"thumbnail": "https://encrypted-tbn2.gstatic.com/shopping?q=tbn:ANd9GcTFEtS8GWRPVvDafLCJqnheugnVYdqrTY2ynzJTflYI_CaiX-kTcvE2pH7H6vXCz-MLCAEYnLclRtaw34-zkOmG3paJqRCVNTeN7pfIBhQauqG4IbqfIePwAg&usqp=CAE"
},
{
"title": "AT&T Samsung Galaxy, A13 Lte, 32gb, Black - Prepaid Smartphone",
"link": "https://www.walmart.com/ip/AT-T-Samsung-Galaxy-A13-LTE-32GB-Black-Prepaid-Smartphone/952665682?wl13=2582&selectedSellerId=0&rct=j&q=&esrc=s&sa=U&ved=0ahUKEwi3yKHT8vf_AhXcJEQIHTs8Dk8QgokFCJ8RKAA&usg=AOvVaw0CHtPxokISjnnhmZaBt3NP",
"price": "$129.00",
"extracted_price": 129,
"stock_information": "In stock",
"thumbnail": "https://encrypted-tbn1.gstatic.com/shopping?q=tbn:ANd9GcT-pPP_uIP6d2AbNnvB9sBOiN_i9V3sa1KKs71mb0z_4oSdRquZLdmyBtSRHFvrk_vxgSmsrgw9NmVHwipKnzqnsXsc6ZPyto64__HNCF-hjS0tjPgu_4TP&usqp=CAE"
},
{
"title": "At&t Samsung A03s, 32gb, Black - Prepaid Smartphone",
"link": "https://www.walmart.com/ip/AT-T-Samsung-A03s-32GB-Black-Prepaid-Smartphone/266313209?wl13=2582&selectedSellerId=0&rct=j&q=&esrc=s&sa=U&ved=0ahUKEwi3yKHT8vf_AhXcJEQIHTs8Dk8QgokFCKERKAA&usg=AOvVaw2rt38mcpiaOzLlr58HrF44",
"price": "$59.88",
"extracted_price": 59.88,
"stock_information": "In stock",
"thumbnail": "https://encrypted-tbn0.gstatic.com/shopping?q=tbn:ANd9GcSrouGDfunfDB5yWzD8C74tvspGB4nQVB9q6cd35fLSFrA9-msmkwBjFehf36Ql2KUHfqQzMtpbNXi22NncQPRWYIitRV8S-XvvHUgeExA&usqp=CAE"
},
{
"title": "Samsung Total by Verizon Samsung Galaxy A03s, 32gb, Black- Prepaid Smartphone [Locked to Total by Verizon]",
"link": "https://www.walmart.com/ip/Total-by-Verizon-Samsung-Galaxy-A03s-32GB-Black-Prepaid-Smartphone-Locked-to-Total-by-Verizon/734772395?wl13=2582&selectedSellerId=0&rct=j&q=&esrc=s&sa=U&ved=0ahUKEwi3yKHT8vf_AhXcJEQIHTs8Dk8QgokFCKMRKAA&usg=AOvVaw2mcWLkr0ZXyjJwNHbwQht2",
"price": "$49.88",
"extracted_price": 49.88,
"stock_information": "In stock",
"thumbnail": "https://encrypted-tbn0.gstatic.com/shopping?q=tbn:ANd9GcT2XEaBYHaAmpBaeQ5AbANIAXheLxb0NBEZPXI-ZfolroJBvQu-kD5fsvjzuJEam7iJjw6o5O4YlwZ4vuD-Vqd4SEbECEDIYN487LqzYBVP3xIlGyMVbdV6&usqp=CAE"
},
{
"title": "Motorola Moto G (2022) 64gb Moonlight Gray AT&T",
"link": "https://www.walmart.com/ip/AT-T-Motorola-Moto-G-5G-64GB-Moonlight-Gray-Prepaid-Smartphone/276456417?wl13=2582&selectedSellerId=0&rct=j&q=&esrc=s&sa=U&ved=0ahUKEwi3yKHT8vf_AhXcJEQIHTs8Dk8QgokFCKURKAA&usg=AOvVaw3FBhdQXb91LR6GFKzFtGy4",
"price": "$99.88",
"extracted_price": 99.88,
"stock_information": "In stock",
"thumbnail": "https://encrypted-tbn0.gstatic.com/shopping?q=tbn:ANd9GcTTfZ3vDhzCZYOfUVRsSDxcpWJkhOZze8zo_-JAgJJIztJsKonBhvnBSJyzTdwMN6aSNfvBCJZiXKLJ9igIR1wfNKVk10iFYsp0vykG9ZMTsz8GBP4AWvaZIg&usqp=CAE"
}
]
},
{
"title": "Best Buy",
"link": "https://www.google.com/search?gl=us&hl=en&q=Best+Buy&ludocid=4675204875206232998&gsas=1&ibp=gwp;0,7&lsig=AB86z5Uaiyb4qn52PLxHs7ugvGOZ&sa=X&ved=0ahUKEwi3yKHT8vf_AhXcJEQIHTs8Dk8QgYkFCKcRKAA",
"address": "531 5th Ave, New York",
"distance": "12.6 miles",
"working_hours": "Today: 10:00 AM - 9:00 PM",
"phone": "(212) 808-0309",
"delivery_options": [
"In‑store pickup"
],
"direction": "https://maps.google.com/maps?gl=us&uule=w+CAIQICIWTmV3IFlvcmssVW5pdGVkIFN0YXRlcw&hl=en&daddr=531+5th+Ave,+New+York,+NY+10017,+United+States",
"items": [
{
"title": "Samsung Galaxy S22 Ultra - 128GB - Burgundy - Verizon",
"link": "https://www.bestbuy.com/site/samsung-galaxy-s22-ultra-128gb-burgundy-verizon/6494451.p?skuId=6494451&ref=212&loc=1&extStoreId=1028&rct=j&q=&esrc=s&sa=U&ved=0ahUKEwi3yKHT8vf_AhXcJEQIHTs8Dk8QgokFCKsRKAA&usg=AOvVaw1wTDr9c3YzxBp0yuzogyuq",
"price": "$0.00 now",
"extracted_price": 0,
"stock_information": "In stock",
"thumbnail": "https://encrypted-tbn0.gstatic.com/shopping?q=tbn:ANd9GcR0FAmzcRwG11hWnlz2M8gvTZm8aWuOZlwm_Xx_CnDrkFuQCGtpSRYKibySQlZi2h7RWdD4EV3KcobiQ3SFWuaseR7_2hKTf80oOkKbBnyw&usqp=CAE"
},
{
"title": "Samsung Galaxy S23 Ultra - 256 GB - Phantom Black - AT&T",
"link": "https://www.bestbuy.com/site/samsung-galaxy-s23-ultra-256gb-phantom-black-at-t/6530819.p?skuId=6530819&ref=212&loc=1&extStoreId=1028&rct=j&q=&esrc=s&sa=U&ved=0ahUKEwi3yKHT8vf_AhXcJEQIHTs8Dk8QgokFCK0RKAA&usg=AOvVaw3oqM6WKXnWlPm2SRd78Bfi",
"price": "$0.00 now",
"extracted_price": 0,
"stock_information": "In stock",
"thumbnail": "https://encrypted-tbn2.gstatic.com/shopping?q=tbn:ANd9GcSyq5YB-Y-fI6eSa7H3pG619yjJETKS9j-36yurwHNRniik0_NXIN447606x86Zz5VjoIvvj2-E3Sch_d_8QZsHrvxsYElNfY_U-n83304X&usqp=CAE"
},
{
"title": "Samsung Galaxy S23 - 128 GB - Phantom Black - AT&T",
"link": "https://www.bestbuy.com/site/samsung-galaxy-s23-128gb-phantom-black-at-t/6530811.p?skuId=6530811&ref=212&loc=1&extStoreId=1028&rct=j&q=&esrc=s&sa=U&ved=0ahUKEwi3yKHT8vf_AhXcJEQIHTs8Dk8QgokFCK8RKAA&usg=AOvVaw0o3pE9pce81wX4co_2zfe1",
"price": "$0.00 now",
"extracted_price": 0,
"stock_information": "In stock",
"thumbnail": "https://encrypted-tbn1.gstatic.com/shopping?q=tbn:ANd9GcQ6XJC3VscrxPqEBZ5cMzDKQ2HufOWrXKQWDtM5vUCe5pbGL4PYII6tIFqmTKCgTm_4DzaV8TGR1mWCYUiUUja09ytI7a5Uma9bt9aAYb8&usqp=CAE"
}
...
]
}
...
]
}
Categories
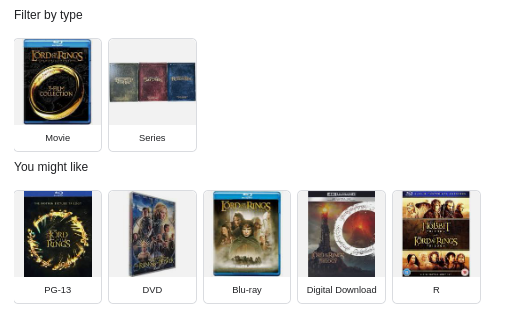
https://www.searchapi.io/api/v1/search?engine=google_shopping&q=LOTR
- Python
- Node
- Ruby
- Java
- Go
- PHP
- Bash
- R
- Kotlin
- Swift
- C#
- C
- C++
- requests
import requests
url = "https://www.searchapi.io/api/v1/search"
params = {
"engine": "google_shopping",
"q": "LOTR"
}
response = requests.get(url, params = params)
print(response.text)
{
"categories": [
{
"type": "Filter by type",
"options": [
{
"title": "Movie",
"link": "https://www.google.com/search?gl=us&hl=en&tbm=shop&q=LOTR&tbs=mr:1,pdtr0:3045649%7C3045651&sa=X&ved=0ahUKEwijkfGO8_f_AhVbFTQIHZn8AroQwQkIjBsoAA",
"thumbnail": "https://encrypted-tbn0.gstatic.com/shopping?q=tbn:ANd9GcSUt5xRGvdk82oP0ELlbpFEXmmtLvXLxcPqQL31CU9ERfZnJp8ygIaHQBH5RkomCj73zNSNuCg&usqp=CAE"
},
{
"title": "Series",
"link": "https://www.google.com/search?gl=us&hl=en&tbm=shop&q=LOTR&tbs=mr:1,pdtr0:3045649%7C3045650&sa=X&ved=0ahUKEwijkfGO8_f_AhVbFTQIHZn8AroQwQkIjxsoAA",
"thumbnail": "https://encrypted-tbn2.gstatic.com/shopping?q=tbn:ANd9GcSwf4o9uAWBl4Gx5tYYTZM6FTd4EA-lYttyIly0sEVwigGdprWO9AZ3LFLrHjBcm_TeFpX4YoE&usqp=CAE"
}
]
},
{
"type": "You might like",
"options": [
{
"title": "PG-13",
"link": "https://www.google.com/search?gl=us&hl=en&tbm=shop&q=LOTR&tbs=mr:1,pdtr0:3057506%7C3057511&sa=X&ved=0ahUKEwijkfGO8_f_AhVbFTQIHZn8AroQwQkIlhsoAA",
"thumbnail": "https://encrypted-tbn3.gstatic.com/shopping?q=tbn:ANd9GcTl9QDfkZb1Rlax7awR8-WmV5z3WgHS-L4WvBC-woU8wTbORmt3QyqUQrw9lgHn_shogOije6c&usqp=CAE"
},
{
"title": "DVD",
"link": "https://www.google.com/search?gl=us&hl=en&tbm=shop&q=LOTR&tbs=mr:1,pdtr0:3045644%7C3045646&sa=X&ved=0ahUKEwijkfGO8_f_AhVbFTQIHZn8AroQwQkImRsoAA",
"thumbnail": "https://encrypted-tbn2.gstatic.com/shopping?q=tbn:ANd9GcSfvSf54wnUHlKrln3T_xLEQuBrq3ieUWAYJqLGpZyFFkZeN6_U5t98IKv4F4igf34jAUPeeUM&usqp=CAE"
},
{
"title": "Blu-ray",
"link": "https://www.google.com/search?gl=us&hl=en&tbm=shop&q=LOTR&tbs=mr:1,pdtr0:3045644%7C3045647&sa=X&ved=0ahUKEwijkfGO8_f_AhVbFTQIHZn8AroQwQkInBsoAA",
"thumbnail": "https://encrypted-tbn1.gstatic.com/shopping?q=tbn:ANd9GcTcK4TTDQXnU7PnC71Uu7Ie1jjAqp_ZTZMBbD0uskAW7M7b81VCPrcXZzwotZyWhiZ_kDqhlV96&usqp=CAE"
},
{
"title": "Digital Download",
"link": "https://www.google.com/search?gl=us&hl=en&tbm=shop&q=LOTR&tbs=mr:1,pdtr0:3045644%7C3120464&sa=X&ved=0ahUKEwijkfGO8_f_AhVbFTQIHZn8AroQwQkInxsoAA",
"thumbnail": "https://encrypted-tbn3.gstatic.com/shopping?q=tbn:ANd9GcShFEL1PG1omKcCnKv8SLpxbE2HuoIHG6zWLn5H-EQjx4k1d_sbcVfZy_AH_w&usqp=CAE"
},
{
"title": "R",
"link": "https://www.google.com/search?gl=us&hl=en&tbm=shop&q=LOTR&tbs=mr:1,pdtr0:3057506%7C3057512&sa=X&ved=0ahUKEwijkfGO8_f_AhVbFTQIHZn8AroQwQkIohsoAA",
"thumbnail": "https://encrypted-tbn2.gstatic.com/shopping?q=tbn:ANd9GcRgLeNumwoqIl-XBOgbRUKnCUpyQQnGOBk6GjdiPbdT8cwNuPStR8hAIkDpaM-B56-jLF53XksV&usqp=CAE"
}
]
}
]
}
Filters
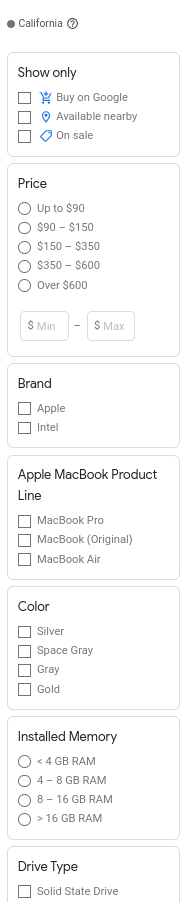
https://www.searchapi.io/api/v1/search?engine=google_shopping&location=New+York%2CUnited+States&q=Macbook
- Python
- Node
- Ruby
- Java
- Go
- PHP
- Bash
- R
- Kotlin
- Swift
- C#
- C
- C++
- requests
import requests
url = "https://www.searchapi.io/api/v1/search"
params = {
"engine": "google_shopping",
"q": "Macbook",
"location": "New York,United States"
}
response = requests.get(url, params = params)
print(response.text)
{
"filters": [
{
"type": "Show only",
"options": [
{
"text": "Buy on Google",
"tbs": "mr:1,buy:g"
},
{
"text": "Available nearby",
"tbs": "mr:1,local_avail:1,ss:55"
},
{
"text": "On sale",
"tbs": "mr:1,sales:1"
}
]
},
{
"type": "Price",
"options": [
{
"text": "Up to $90",
"tbs": "mr:1,price:1,ppr_max:90"
},
{
"text": "$90 – $150",
"tbs": "mr:1,price:1,ppr_min:90,ppr_max:150"
},
{
"text": "$150 – $350",
"tbs": "mr:1,price:1,ppr_min:150,ppr_max:350"
},
{
"text": "$350 – $600",
"tbs": "mr:1,price:1,ppr_min:350,ppr_max:600"
},
{
"text": "Over $600",
"tbs": "mr:1,price:1,ppr_min:600"
},
{}
]
},
{
"type": "Brand",
"options": [
{
"text": "Apple",
"tbs": "mr:1,pdtr0:703960%7C703973"
},
{
"text": "Intel",
"tbs": "mr:1,pdtr0:703960%7C1043386"
}
]
},
{
"type": "Apple MacBook Product Line",
"options": [
{
"text": "MacBook Pro",
"tbs": "mr:1,pdtr0:946505%7C946506"
},
{
"text": "MacBook (Original)",
"tbs": "mr:1,pdtr0:946505%7C972425"
},
{
"text": "MacBook Air",
"tbs": "mr:1,pdtr0:946505%7C946507"
}
]
},
{
"type": "Color",
"options": [
{
"text": "Silver",
"tbs": "mr:1,pdtr0:1716119%7C1716122"
},
{
"text": "Space Gray",
"tbs": "mr:1,pdtr0:1716119%7C1716123"
},
{
"text": "Gray",
"tbs": "mr:1,pdtr0:1716119%7C1716124"
},
{
"text": "Gold",
"tbs": "mr:1,pdtr0:1716119%7C1716128"
}
]
},
{
"type": "Installed Memory",
"options": [
{
"text": "< 4 GB RAM",
"tbs": "mr:1,pdtr0:1020720%7C%244.0"
},
{
"text": "4 – 8 GB RAM",
"tbs": "mr:1,pdtr0:1020720%7C4.0%248.0"
}
...
]
}
...
]
}
Related Results
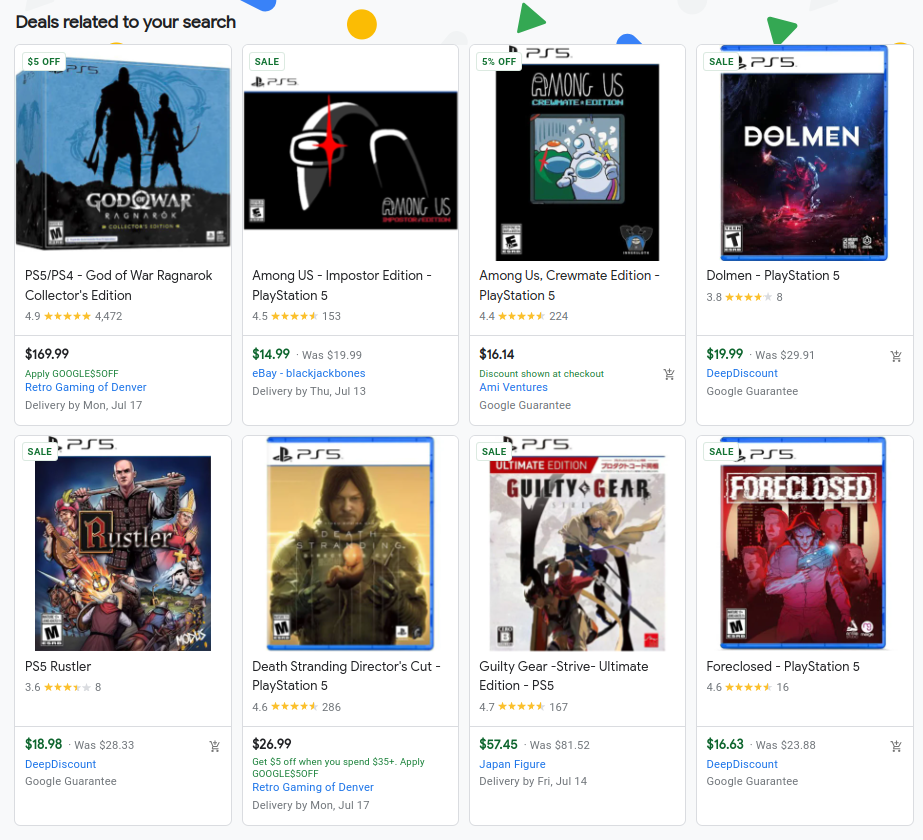
https://www.searchapi.io/api/v1/search?engine=google_shopping&location=Miami-Dade+County%2CFlorida%2CUnited+States&q=PS5
- Python
- Node
- Ruby
- Java
- Go
- PHP
- Bash
- R
- Kotlin
- Swift
- C#
- C
- C++
- requests
import requests
url = "https://www.searchapi.io/api/v1/search"
params = {
"engine": "google_shopping",
"q": "PS5",
"location": "Miami-Dade County,Florida,United States"
}
response = requests.get(url, params = params)
print(response.text)
{
"related_results": [
{
"position": 1,
"product_id": "2085922572895778480",
"title": "PS5/PS4 - God of War Ragnarok Collector's Edition",
"link": "https://retrogamingofdenver.com/products/god-of-war-ragnarok-collectors-edition-playstation-5-new?variant=43815537017073¤cy=USD&utm_medium=product_sync&utm_source=google&utm_content=sag_organic&utm_campaign=sag_organic&srsltid=ASuE1wSenCNJ90RSI39L9OT1Fb1ZGLJL7sffKcEl8QRyU-EmbG-fVLNDYSM&rct=j&q=&esrc=s&sa=U&ved=0ahUKEwiOo_--7_f_AhWYLUQIHdFuABYQguUECJAZ&usg=AOvVaw0AxnPaaRUDjNiYlQG9ZZMX",
"product_link": "https://www.google.com/shopping/product/2085922572895778480?q=PS5&ie=UTF-8&uule=w+CAIQICIYQ2FsaWZvcm5pYSxVbml0ZWQgU3RhdGVz&oq=PS5&gl=us&hl=en&prds=pid:2502665397428699522,rsk:PC_6528732777392445047&sa=X&ved=0ahUKEwiOo_--7_f_AhWYLUQIHdFuABYQ8gIIihkoAA",
"source": "Retro Gaming of Denver",
"discount": "Apply GOOGLE$5OFF",
"price": "$169.99",
"extracted_price": 169.99,
"rating": 4.9,
"reviews": 4472,
"tag": "$5 OFF",
"delivery": "Delivery by Mon, Jul 17",
"thumbnail": "https://encrypted-tbn1.gstatic.com/shopping?q=tbn:ANd9GcTo4AdcLGHku3_Uky04DtqpeEsAW0yFzw6uSBXOEhK4BIbzpF4J32M6TPer3gx2Lpj49XGbjHPLLoRMeLVvX1VwbpEHSkVJXopTfWBwqBQRdihJXwwkhUkM&usqp=CAE"
},
{
"position": 2,
"product_id": "5395677433014263836",
"title": "Among US - Impostor Edition - PlayStation 5",
"link": "https://www.ebay.com/itm/266266230370?chn=ps&mkevt=1&mkcid=28&srsltid=ASuE1wRwrOmYXmujbiu333n9BmkaP324XHHYV5C0V9DFoCmB-7FwBxZ8jRg&rct=j&q=&esrc=s&sa=U&ved=0ahUKEwiOo_--7_f_AhWYLUQIHdFuABYQguUECKEZ&usg=AOvVaw0tZfmYMWAKXwPHygv4JWH9",
"product_link": "https://www.google.com/shopping/product/5395677433014263836?q=PS5&ie=UTF-8&uule=w+CAIQICIYQ2FsaWZvcm5pYSxVbml0ZWQgU3RhdGVz&oq=PS5&gl=us&hl=en&prds=pid:8024874914570755010,rsk:PC_13556248843592479408&sa=X&ved=0ahUKEwiOo_--7_f_AhWYLUQIHdFuABYQ8gIImxkoAA",
"source": "eBay - blackjackbones",
"price": "$14.99",
"extracted_price": 14.99,
"original_price": "Was $19.99",
"extracted_original_price": 19.99,
"rating": 4.5,
"reviews": 153,
"tag": "SALE",
"delivery": "Delivery by Thu, Jul 13",
"thumbnail": "https://encrypted-tbn3.gstatic.com/shopping?q=tbn:ANd9GcRrURHiP-Kf_BPgBZrzBUXaVwJnK0GuJTHu0RHqrzWCrPLYQUe4jWsVewYRunWhPOXOCYFj_RsX0yiR3YPGHSrHyJg838OQDTJqAJNfCfPlJLuLuN3fzOXIYQ&usqp=CAE"
},
{
"position": 3,
"product_id": "7416062647395280475",
"title": "Among Us, Crewmate Edition - PlayStation 5",
"link": "#&rct=j&q=&esrc=s&sa=U&ved=0ahUKEwiOo_--7_f_AhWYLUQIHdFuABYQguUECLMZ&usg=AOvVaw3Fr-lfi2zR_kPjnk8bGdX6",
"product_link": "https://www.google.com/shopping/product/7416062647395280475?q=PS5&ie=UTF-8&uule=w+CAIQICIYQ2FsaWZvcm5pYSxVbml0ZWQgU3RhdGVz&oq=PS5&gl=us&hl=en&prds=pid:15810759107001688362,rsk:PC_16050490641271646149&sa=X&ved=0ahUKEwiOo_--7_f_AhWYLUQIHdFuABYQ8gIIrhkoAA",
"source": "Ami Ventures",
"discount": "Discount shown at checkout",
"price": "$16.14",
"extracted_price": 16.14,
"rating": 4.4,
"reviews": 224,
"tag": "5% OFF",
"delivery": "Google Guarantee",
"thumbnail": "https://encrypted-tbn3.gstatic.com/shopping?q=tbn:ANd9GcS9pTGcSXuVhuj438UihZRTT5q6fyj65JfDL9EQjfEkNRRMaHorLqyHQnwsfj3wvOnpclfCqU6dznZYpuRAm_6Op3oowA_Bmo-oyCaNwry3-HtQKWyd1HIF&usqp=CAE"
},
{
"position": 4,
"product_id": "14739812013792815258",
"title": "Dolmen - PlayStation 5",
"link": "https://www.deepdiscount.com/goog/810086920037&rct=j&q=&esrc=s&sa=U&ved=0ahUKEwiOo_--7_f_AhWYLUQIHdFuABYQguUECMUZ&usg=AOvVaw2oB-lbnQ3CvBFGpuoLn2-V",
"product_link": "https://www.google.com/shopping/product/14739812013792815258?q=PS5&ie=UTF-8&uule=w+CAIQICIYQ2FsaWZvcm5pYSxVbml0ZWQgU3RhdGVz&oq=PS5&gl=us&hl=en&prds=pid:1765706353169471088,rsk:CID_14739812013792815258&sa=X&ved=0ahUKEwiOo_--7_f_AhWYLUQIHdFuABYQ8gIIvxkoAA",
"source": "DeepDiscount",
"price": "$19.99",
"extracted_price": 19.99,
"original_price": "Was $29.91",
"extracted_original_price": 29.91,
"rating": 3.8,
"reviews": 8,
"tag": "SALE",
"delivery": "Google Guarantee",
"thumbnail": "https://encrypted-tbn0.gstatic.com/shopping?q=tbn:ANd9GcS714yXYtxsDVEFOxZjlvxo1HV8vC7pqMK3IAgsA0ESWzfegEaMkuvvvQGMhz-RlOtVko0nHlQtFmucl_yZVCuJxIpCj-jb_9DRTGPrHMwL&usqp=CAE"
},
{
"position": 5,
"product_id": "7007321996971862281",
"title": "PS5 Rustler",
"link": "https://www.deepdiscount.com/goog/814290017088&rct=j&q=&esrc=s&sa=U&ved=0ahUKEwiOo_--7_f_AhWYLUQIHdFuABYQguUECNYZ&usg=AOvVaw14tAHkZpM_1oEwZQN06Q06",
"product_link": "https://www.google.com/shopping/product/7007321996971862281?q=PS5&ie=UTF-8&uule=w+CAIQICIYQ2FsaWZvcm5pYSxVbml0ZWQgU3RhdGVz&oq=PS5&gl=us&hl=en&prds=pid:5207733865824988147,rsk:PC_3634866181820049462&sa=X&ved=0ahUKEwiOo_--7_f_AhWYLUQIHdFuABYQ8gII0BkoAA",
"source": "DeepDiscount",
"price": "$18.98",
"extracted_price": 18.98,
"original_price": "Was $28.33",
"extracted_original_price": 28.33,
"rating": 3.6,
"reviews": 8,
"tag": "SALE",
"delivery": "Google Guarantee",
"thumbnail": "https://encrypted-tbn2.gstatic.com/shopping?q=tbn:ANd9GcQ6UVYn5Kt1l4eMpUTb4038RZCV0Hy_5T5OHSveYEcEoj9wmo8de1DvpZ9OOVMw-tOc8u-jqwk65ofm-R-MOBFwcGa8szI0zshCr-AR1jpf-RfyJCGcY9v7yg&usqp=CAE"
},
{
"position": 6,
"product_id": "1741084946725213571",
"title": "Death Stranding Director's Cut - PlayStation 5",
"link": "https://retrogamingofdenver.com/products/death-stranding-director-s-cut-playstation-5?variant=42583669637361¤cy=USD&utm_medium=product_sync&utm_source=google&utm_content=sag_organic&utm_campaign=sag_organic&srsltid=ASuE1wRjcDIdsrdbET9Fe7kXx_ZCZ7gSxa39-LIWvG7hfeGWTc3p9SExa7w&rct=j&q=&esrc=s&sa=U&ved=0ahUKEwiOo_--7_f_AhWYLUQIHdFuABYQguUECOkZ&usg=AOvVaw2nqgeX7exuZpLyiChhp-qT",
"product_link": "https://www.google.com/shopping/product/1741084946725213571?q=PS5&ie=UTF-8&uule=w+CAIQICIYQ2FsaWZvcm5pYSxVbml0ZWQgU3RhdGVz&oq=PS5&gl=us&hl=en&prds=pid:8920675167380856439,rsk:PC_4198874846042996551&sa=X&ved=0ahUKEwiOo_--7_f_AhWYLUQIHdFuABYQ8gII4xkoAA",
"source": "Retro Gaming of Denver",
"discount": "Get $5 off when you spend $35+. Apply GOOGLE$5OFF",
"price": "$26.99",
"extracted_price": 26.99,
"rating": 4.6,
"reviews": 286,
"delivery": "Delivery by Mon, Jul 17",
"thumbnail": "https://encrypted-tbn3.gstatic.com/shopping?q=tbn:ANd9GcQv6ILzNxUxAcC6O6_QHo-ihg70LbpL1qfT1IS8wbUUTrrPb168Hklb9m3Z9gK7vI79ZfLqHjDme8gm1X7igAPHtWTelB72Ky7UKaFKsXZCS_sfyLbthd-g&usqp=CAE"
}
...
]
}
Related Searches
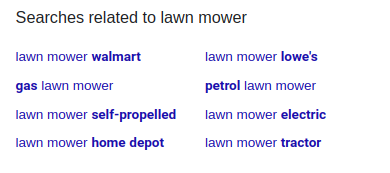
https://www.searchapi.io/api/v1/search?engine=google_shopping&location=Miami-Dade+County%2CFlorida%2CUnited+States&q=lawn+mower
- Python
- Node
- Ruby
- Java
- Go
- PHP
- Bash
- R
- Kotlin
- Swift
- C#
- C
- C++
- requests
import requests
url = "https://www.searchapi.io/api/v1/search"
params = {
"engine": "google_shopping",
"q": "lawn mower",
"location": "Miami-Dade County,Florida,United States"
}
response = requests.get(url, params = params)
print(response.text)
{
"related_searches": [
{
"query": "lawn mower walmart",
"link": "https://www.google.com/search?hl=en&gl=us&tbm=shop&q=lawn+mower+walmart&sa=X&ved=0ahUKEwiShLKP5Y2AAxXuSTABHZJhASYQ2wsIwRg"
},
{
"query": "gas lawn mower",
"link": "https://www.google.com/search?hl=en&gl=us&tbm=shop&q=gas+lawn+mower&sa=X&ved=0ahUKEwiShLKP5Y2AAxXuSTABHZJhASYQ2wsIwxg"
},
{
"query": "lawn mower self-propelled",
"link": "https://www.google.com/search?hl=en&gl=us&tbm=shop&q=lawn+mower+self-propelled&sa=X&ved=0ahUKEwiShLKP5Y2AAxXuSTABHZJhASYQ2wsIxRg"
},
{
"query": "lawn mower home depot",
"link": "https://www.google.com/search?hl=en&gl=us&tbm=shop&q=lawn+mower+home+depot&sa=X&ved=0ahUKEwiShLKP5Y2AAxXuSTABHZJhASYQ2wsIxxg"
},
{
"query": "lawn mower lowe's",
"link": "https://www.google.com/search?hl=en&gl=us&tbm=shop&q=lawn+mower+lowe%27s&sa=X&ved=0ahUKEwiShLKP5Y2AAxXuSTABHZJhASYQ2wsIyRg"
},
{
"query": "petrol lawn mower",
"link": "https://www.google.com/search?hl=en&gl=us&tbm=shop&q=petrol+lawn+mower&sa=X&ved=0ahUKEwiShLKP5Y2AAxXuSTABHZJhASYQ2wsIyxg"
},
{
"query": "lawn mower electric",
"link": "https://www.google.com/search?hl=en&gl=us&tbm=shop&q=lawn+mower+electric&sa=X&ved=0ahUKEwiShLKP5Y2AAxXuSTABHZJhASYQ2wsIzRg"
},
{
"query": "lawn mower tractor",
"link": "https://www.google.com/search?hl=en&gl=us&tbm=shop&q=lawn+mower+tractor&sa=X&ved=0ahUKEwiShLKP5Y2AAxXuSTABHZJhASYQ2wsIzxg"
}
]
}